mirror of
git://xwords.git.sourceforge.net/gitroot/xwords/xwords
synced 2025-01-10 05:26:10 +01:00
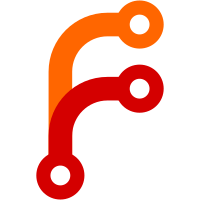
Before writing to storage, ask permission. This adds basic ability to query and route the OS's callback back to the code that asked.
238 lines
7.1 KiB
Groovy
238 lines
7.1 KiB
Groovy
def VARIANT_NAME = 'xw4'
|
|
def INITIAL_CLIENT_VERS = 6
|
|
def CHAT_ENABLED = true
|
|
def LIBS_DEBUG = 'libs-debug'
|
|
def LIBS_RELEASE = 'libs-release'
|
|
def VERSION_CODE_BASE = 1
|
|
def VARIANTS = [ "Xw4", "Xw4dbg" ]
|
|
def BUILDS = [ "Debug", "Release" ]
|
|
|
|
apply plugin: 'com.android.application'
|
|
|
|
dependencies {
|
|
compile files('../libs/gcm.jar')
|
|
// compile files('../libs/android-support-v13.jar')
|
|
compile 'com.android.support:support-v4:23.0.0'
|
|
}
|
|
|
|
android {
|
|
compileSdkVersion 23
|
|
buildToolsVersion "23.0.2"
|
|
defaultConfig {
|
|
minSdkVersion 7
|
|
targetSdkVersion 23
|
|
}
|
|
|
|
// Rename all output artifacts to include version information
|
|
// applicationVariants.all { variant ->
|
|
// renameArtifact(variant)
|
|
// variant.buildConfigField "String", "FIELD_NAME", "\"my String\""
|
|
// }
|
|
|
|
flavorDimensions "variant"//, "abi"
|
|
productFlavors {
|
|
xw4 {
|
|
dimension "variant"
|
|
applicationId "org.eehouse.android.xw4"
|
|
}
|
|
xw4dbg {
|
|
dimension "variant"
|
|
applicationId "org.eehouse.android.xw4dbg"
|
|
}
|
|
|
|
// WARNING: "all" breaks things. Seems to be a keyword. Need
|
|
// to figure out how to express include-all-abis
|
|
// all {
|
|
// dimension "abi"
|
|
// versionCode 0 + VERSION_CODE_BASE
|
|
// }
|
|
// armeabi {
|
|
// dimension "abi"
|
|
// versionCode 1 + VERSION_CODE_BASE
|
|
// }
|
|
// x86 {
|
|
// dimension "abi"
|
|
// versionCode 2 + VERSION_CODE_BASE
|
|
// }
|
|
// armeabiv7a {
|
|
// dimension "abi"
|
|
// versionCode 3 + VERSION_CODE_BASE
|
|
// }
|
|
|
|
}
|
|
|
|
signingConfigs {
|
|
release {
|
|
storeFile file(System.getenv("HOME") + "/.keystore")
|
|
keyAlias "mykey"
|
|
|
|
// These two lines make gradle believe that the signingConfigs
|
|
// section is complete. Without them, tasks like installRelease
|
|
// will not be available!
|
|
storePassword "notReal"
|
|
keyPassword "notReal"
|
|
}
|
|
}
|
|
|
|
buildTypes {
|
|
release {
|
|
signingConfig signingConfigs.release
|
|
debuggable false
|
|
minifyEnabled true
|
|
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro'
|
|
}
|
|
debug {
|
|
debuggable true
|
|
// This doesn't work on marshmallow: duplicate permission error
|
|
// applicationIdSuffix ".debug"
|
|
}
|
|
}
|
|
|
|
sourceSets {
|
|
// Use symlinks instead of setting non-conventional
|
|
// directories here. AS doesn't respect what's set here: it'll
|
|
// compile, but post-install app launch and source-level
|
|
// debugging don't work.
|
|
release {
|
|
jniLibs.srcDir "../$LIBS_RELEASE"
|
|
}
|
|
debug {
|
|
jniLibs.srcDir "../$LIBS_DEBUG"
|
|
}
|
|
}
|
|
|
|
lintOptions {
|
|
abortOnError false
|
|
}
|
|
|
|
def gitrev = "git describe --tags".execute().text.trim()
|
|
applicationVariants.all { variant ->
|
|
variant.outputs.each { output ->
|
|
output.outputFile =
|
|
new File(output.outputFile.parent,
|
|
output.outputFile.name.replace(".apk", "-${gitrev}.apk"))
|
|
}
|
|
}
|
|
}
|
|
|
|
// Prevent release builds. They haven't been tested, and now that
|
|
// f-droid has been releasing them they're KNOWN not to work.
|
|
android.applicationVariants.all { variant ->
|
|
if ( variant.name.endsWith("Release") ) {
|
|
String NAME = "check" + variant.name.capitalize() + "Manifest"
|
|
task "$NAME"(overwrite: true) << {
|
|
throw new RuntimeException('<<<<< Release builds should not be built '
|
|
+ 'using gradle! Please use ant for now. >>>>>');
|
|
}
|
|
}
|
|
}
|
|
|
|
dependencies {
|
|
compile fileTree(dir: 'libs', include: ['*.jar'])
|
|
}
|
|
|
|
task genVers(type: Exec) {
|
|
workingDir '../'
|
|
commandLine '../scripts/genvers.sh', '--variant', VARIANT_NAME,
|
|
'--client-vers', INITIAL_CLIENT_VERS,
|
|
'--vers-outfile', "assets/gitvers.txt"
|
|
}
|
|
|
|
task mkImages(type: Exec) {
|
|
workingDir '../'
|
|
commandLine '../scripts/mkimages.sh'
|
|
}
|
|
|
|
task copyStrings(type: Exec) {
|
|
workingDir '../'
|
|
commandLine '../scripts/copy-strings.py'
|
|
}
|
|
|
|
task ndkSetup(type: Exec) {
|
|
workingDir '../'
|
|
commandLine "../scripts/ndksetup.sh"
|
|
}
|
|
|
|
task myPreBuild(dependsOn: ['genVers', 'ndkSetup', 'mkImages', 'copyStrings', 'mkXml']) {
|
|
}
|
|
preBuild.dependsOn myPreBuild
|
|
|
|
task ndkBuildDebug(type: Exec) {
|
|
workingDir '../'
|
|
commandLine '../scripts/ndkbuild.sh', '-j3', "CHAT_ENABLED=$CHAT_ENABLED",
|
|
'BUILD_TARGET=debug', "INITIAL_CLIENT_VERS=$INITIAL_CLIENT_VERS",
|
|
"VARIANT=$VARIANT_NAME", "NDK_LIBS_OUT=$LIBS_DEBUG", 'NDK_OUT=./obj-debug'
|
|
}
|
|
|
|
task ndkBuildRelease(type: Exec) {
|
|
workingDir '../'
|
|
commandLine '../scripts/ndkbuild.sh', '-j3', "CHAT_ENABLED=$CHAT_ENABLED",
|
|
'BUILD_TARGET=release', "INITIAL_CLIENT_VERS=$INITIAL_CLIENT_VERS",
|
|
"VARIANT=$VARIANT_NAME", "NDK_LIBS_OUT=$LIBS_RELEASE", 'NDK_OUT=./obj-release'
|
|
}
|
|
|
|
task mkXml(type: Exec) {
|
|
workingDir '../'
|
|
commandLine '../scripts/mk_xml.py', '-o',
|
|
"src/org/eehouse/android/$VARIANT_NAME/loc/LocIDsData.java",
|
|
'-t', "debug", '-v', "$VARIANT_NAME"
|
|
}
|
|
|
|
afterEvaluate {
|
|
VARIANTS.each { VARIANT ->
|
|
String compileTask = "compile${VARIANT}ReleaseNdk"
|
|
tasks.getByName(compileTask).dependsOn ndkBuildRelease
|
|
compileTask = "compile${VARIANT}DebugNdk"
|
|
tasks.getByName(compileTask).dependsOn ndkBuildDebug
|
|
}
|
|
}
|
|
|
|
task askForPassword << {
|
|
def password = System.getenv("ANDROID_KEY_PASS")
|
|
if (null == password || 0 == password.length()) {
|
|
if ( null != System.console() ) {
|
|
password = new String(System.console()
|
|
.readPassword("ANDROID_KEY_PASS not set; "
|
|
+ "Keystore password: "))
|
|
} else {
|
|
password = null
|
|
println( "ANDROID_KEY_PASS not set and no console; " )
|
|
println( "sign it yerself later. (Or you might try" )
|
|
println( " running gradlew with the --no-daemon flag)" )
|
|
}
|
|
}
|
|
|
|
if ( null != password ) {
|
|
android.signingConfigs.release.storePassword = password
|
|
android.signingConfigs.release.keyPassword = password
|
|
}
|
|
}
|
|
|
|
tasks.whenTaskAdded { theTask ->
|
|
if (theTask.name.equals("packageRelease")) {
|
|
theTask.dependsOn "askForPassword"
|
|
}
|
|
}
|
|
|
|
// def getVersionName() {
|
|
// try {
|
|
// def stdout = new ByteArrayOutputStream()
|
|
// exec {
|
|
// commandLine 'git', 'describe', '--dirty'
|
|
// standardOutput = stdout
|
|
// }
|
|
// return stdout.toString().trim()
|
|
// }
|
|
// catch (ignored) {
|
|
// return null;
|
|
// }
|
|
// }
|
|
|
|
// def renameArtifact(variant) {
|
|
// variant.outputs.each { output ->
|
|
// def name = String.format( "XWords4-%s-%s.apk", variant.name,
|
|
// getVersionName() )
|
|
// output.outputFile = new File( (String)output.outputFile.parent,
|
|
// (String)name )
|
|
// }
|
|
// }
|