mirror of
git://xwords.git.sourceforge.net/gitroot/xwords/xwords
synced 2025-01-03 23:04:08 +01:00
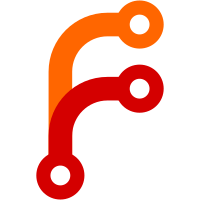
I'm wrestling with how to have concurrent tabs open. They can't be on the same game because both can't connect using same MQTT dev id (and they'd quickly get the saved state screwed up.) Only problem is sometimes a tab in a new window doesn't have access to localstorage and so is able to connect on its own. But later that state isn't reachable any more: relaunch firefox and you get back the to the older state.
210 lines
8 KiB
HTML
210 lines
8 KiB
HTML
<!doctype html>
|
|
<html lang="en-us">
|
|
<head>
|
|
<meta charset="utf-8">
|
|
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
|
|
<title>CrossWords webapp prototype</title>
|
|
<style>
|
|
.emscripten { padding-right: 0; margin-left: auto; margin-right: auto; display: block; }
|
|
textarea.emscripten { font-family: monospace; width: 80%; }
|
|
body.centered { text-align: center; }
|
|
div.emscripten { text-align: center; }
|
|
div.emscripten_border { border: 1px solid black; }
|
|
/* the canvas *must not* have any border or padding, or mouse coords will be wrong */
|
|
canvas.emscripten { border: 0px none; background-color: black; }
|
|
|
|
div.buttonRow {
|
|
text-align: center;
|
|
padding: 7px;
|
|
}
|
|
|
|
.spinner {
|
|
height: 50px;
|
|
width: 50px;
|
|
margin: 0px auto;
|
|
-webkit-animation: rotation .8s linear infinite;
|
|
-moz-animation: rotation .8s linear infinite;
|
|
-o-animation: rotation .8s linear infinite;
|
|
animation: rotation 0.8s linear infinite;
|
|
border-left: 10px solid rgb(0,150,240);
|
|
border-right: 10px solid rgb(0,150,240);
|
|
border-bottom: 10px solid rgb(0,150,240);
|
|
border-top: 10px solid rgb(100,0,200);
|
|
border-radius: 100%;
|
|
background-color: rgb(200,100,250);
|
|
}
|
|
@-webkit-keyframes rotation {
|
|
from {-webkit-transform: rotate(0deg);}
|
|
to {-webkit-transform: rotate(360deg);}
|
|
}
|
|
@-moz-keyframes rotation {
|
|
from {-moz-transform: rotate(0deg);}
|
|
to {-moz-transform: rotate(360deg);}
|
|
}
|
|
@-o-keyframes rotation {
|
|
from {-o-transform: rotate(0deg);}
|
|
to {-o-transform: rotate(360deg);}
|
|
}
|
|
@keyframes rotation {
|
|
from {transform: rotate(0deg);}
|
|
to {transform: rotate(360deg);}
|
|
}
|
|
|
|
#nbalert {
|
|
display: none;
|
|
position: absolute;
|
|
text-align: left;
|
|
z-index: 10000;
|
|
white-space: pre-wrap;
|
|
left: 33%;
|
|
top: 250px;
|
|
width: 33%;
|
|
background-color: #FFF;
|
|
min-width: 217px;
|
|
border-radius: 10px 10px;
|
|
border: solid;
|
|
border-width: 1px;
|
|
border-color: #000;
|
|
vertical-align: top;
|
|
padding: 10px;
|
|
}
|
|
|
|
</style>
|
|
</head>
|
|
<body class="centered">
|
|
<hr/>
|
|
<figure style="overflow:visible;" id="spinner"><div class="spinner"></div><center style="margin-top:0.5em"><strong>emscripten</strong></center></figure>
|
|
<div class="emscripten" id="status">Downloading...</div>
|
|
<div class="emscripten">
|
|
<progress value="0" max="100" id="progress" hidden=1></progress>
|
|
</div>
|
|
<div id='nbalert'></div>
|
|
<div class="emscripten_border">
|
|
<canvas class="emscripten" id="canvas" oncontextmenu="event.preventDefault()" tabindex=-1></canvas>
|
|
</div>
|
|
<div class='buttonRow'>
|
|
<button id="hintdown", onclick="callButton(this);">Prev Hint</button>
|
|
<button id="hintup", onclick="callButton(this);">Next Hint</button>
|
|
<button id="trade", onclick="callButton(this);">Trade</button>
|
|
<button id="commit", onclick="callButton(this);">Commit</button>
|
|
<button id="flip", onclick="callButton(this);">Flip</button>
|
|
<button id="redo", onclick="callButton(this);">Un/Redo</button>
|
|
<button id="vals", onclick="callButton(this);">Vals</button>
|
|
</div>
|
|
<div>
|
|
<span>Player 1</span>
|
|
<input type="checkbox" id="player0Checked">Is Robot</input>
|
|
</div>
|
|
<div>
|
|
<span>Player 2</span>
|
|
<input type="checkbox" id="player1Checked">Is Robot</input>
|
|
</div>
|
|
<div>
|
|
<button type="button" onclick="callNewGame();">New Local Game</button>
|
|
</div>
|
|
<hr/>
|
|
|
|
<div><span>MQTT Dev ID:</span><span id="mqtt_span"></span></div>
|
|
<div><span>MQTT Status:</span><span id="mqtt_status">Unconnected</span></div>
|
|
<div>
|
|
<button type="button" onclick="localStorage.clear();">Clear Local Storage</button>
|
|
</div>
|
|
<hr/>
|
|
<div class="emscripten">
|
|
<input type="checkbox" id="resize">Resize canvas
|
|
<input type="checkbox" id="pointerLock" checked>Lock/hide mouse pointer
|
|
|
|
<input type="button" value="Fullscreen" onclick="Module.requestFullscreen(document.getElementById('pointerLock').checked,
|
|
document.getElementById('resize').checked)">
|
|
</div>
|
|
|
|
<hr/>
|
|
<textarea class="emscripten" id="output" rows="8"></textarea>
|
|
<hr>
|
|
<script type='text/javascript'>
|
|
var statusElement = document.getElementById('status');
|
|
var progressElement = document.getElementById('progress');
|
|
var spinnerElement = document.getElementById('spinner');
|
|
|
|
let arguments = window.location.search.replaceAll('?', '').trim().split('&');
|
|
|
|
var Module = {
|
|
preRun: [],
|
|
postRun: [],
|
|
arguments: arguments,
|
|
print: (function() {
|
|
var element = document.getElementById('output');
|
|
if (element) element.value = ''; // clear browser cache
|
|
return function(text) {
|
|
if (arguments.length > 1) text = Array.prototype.slice.call(arguments).join(' ');
|
|
// These replacements are necessary if you render to raw HTML
|
|
//text = text.replace(/&/g, "&");
|
|
//text = text.replace(/</g, "<");
|
|
//text = text.replace(/>/g, ">");
|
|
//text = text.replace('\n', '<br>', 'g');
|
|
console.log(text);
|
|
if (element) {
|
|
element.value += text + "\n";
|
|
element.scrollTop = element.scrollHeight; // focus on bottom
|
|
}
|
|
};
|
|
})(),
|
|
printErr: function(text) {
|
|
if (arguments.length > 1) text = Array.prototype.slice.call(arguments).join(' ');
|
|
console.error(text);
|
|
},
|
|
canvas: (function() {
|
|
var canvas = document.getElementById('canvas');
|
|
|
|
// As a default initial behavior, pop up an alert when webgl context is lost. To make your
|
|
// application robust, you may want to override this behavior before shipping!
|
|
// See http://www.khronos.org/registry/webgl/specs/latest/1.0/#5.15.2
|
|
canvas.addEventListener("webglcontextlost", function(e) { alert('WebGL context lost. You will need to reload the page.'); e.preventDefault(); }, false);
|
|
|
|
return canvas;
|
|
})(),
|
|
setStatus: function(text) {
|
|
if (!Module.setStatus.last) Module.setStatus.last = { time: Date.now(), text: '' };
|
|
if (text === Module.setStatus.last.text) return;
|
|
var m = text.match(/([^(]+)\((\d+(\.\d+)?)\/(\d+)\)/);
|
|
var now = Date.now();
|
|
if (m && now - Module.setStatus.last.time < 30) return; // if this is a progress update, skip it if too soon
|
|
Module.setStatus.last.time = now;
|
|
Module.setStatus.last.text = text;
|
|
if (m) {
|
|
text = m[1];
|
|
progressElement.value = parseInt(m[2])*100;
|
|
progressElement.max = parseInt(m[4])*100;
|
|
progressElement.hidden = false;
|
|
spinnerElement.hidden = false;
|
|
} else {
|
|
progressElement.value = null;
|
|
progressElement.max = null;
|
|
progressElement.hidden = true;
|
|
if (!text) spinnerElement.hidden = true;
|
|
}
|
|
statusElement.innerHTML = text;
|
|
},
|
|
totalDependencies: 0,
|
|
monitorRunDependencies: function(left) {
|
|
this.totalDependencies = Math.max(this.totalDependencies, left);
|
|
Module.setStatus(left ? 'Preparing... (' + (this.totalDependencies-left) + '/' + this.totalDependencies + ')' : 'All downloads complete.');
|
|
}
|
|
};
|
|
Module.setStatus('Downloading...');
|
|
window.onerror = function() {
|
|
Module.setStatus('Exception thrown, see JavaScript console');
|
|
spinnerElement.style.display = 'none';
|
|
Module.setStatus = function(text) {
|
|
if (text) Module.printErr('[post-exception status] ' + text);
|
|
};
|
|
};
|
|
</script>
|
|
<script>
|
|
let script = document.createElement('script');
|
|
script.src = "xwutils.js";
|
|
document.body.appendChild(script);
|
|
</script>
|
|
{{{ SCRIPT }}}
|
|
</body>
|
|
</html>
|