mirror of
git://xwords.git.sourceforge.net/gitroot/xwords/xwords
synced 2024-12-29 10:26:36 +01:00
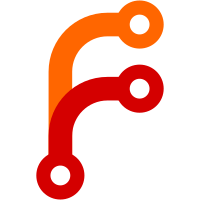
2509 2513 2514 2515 2516 2517 2518 2519 2521 2522 2523 2524 2525 2526 2527 2528 2529 2530 2531 2532 2534 2535 2536 2537 2538 2539 2540 2541 2542 2543 and 2544 from trunk with the goal of adding localization support to this branch so that it can be released before all the networking stuff on trunk is debugged.
102 lines
3.3 KiB
C
Executable file
102 lines
3.3 KiB
C
Executable file
/* -*-mode: C; fill-column: 77; c-basic-offset: 4; -*- */
|
|
/*
|
|
* Copyright 2002-2004 by Eric House (xwords@eehouse.org). All rights reserved.
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License
|
|
* as published by the Free Software Foundation; either version 2
|
|
* of the License, or (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
|
|
*/
|
|
|
|
#ifndef _CEUTIL_H_
|
|
#define _CEUTIL_H_
|
|
|
|
#include "stdafx.h"
|
|
#include "cemain.h"
|
|
|
|
void ceSetDlgItemText( HWND hDlg, XP_U16 id, const XP_UCHAR* buf );
|
|
void ceGetDlgItemText( HWND hDlg, XP_U16 id, XP_UCHAR* buf, XP_U16* bLen );
|
|
|
|
void ceSetDlgItemNum( HWND hDlg, XP_U16 id, XP_S32 num );
|
|
XP_S32 ceGetDlgItemNum( HWND hDlg, XP_U16 id );
|
|
|
|
void ceSetDlgItemFileName( HWND hDlg, XP_U16 id, XP_UCHAR* buf );
|
|
|
|
void positionDlg( HWND hDlg );
|
|
|
|
void ce_selectAndShow( HWND hDlg, XP_U16 resID, XP_U16 index );
|
|
|
|
void ceShowOrHide( HWND hDlg, XP_U16 resID, XP_Bool visible );
|
|
void ceEnOrDisable( HWND hDlg, XP_U16 resID, XP_Bool visible );
|
|
|
|
XP_Bool ceGetChecked( HWND hDlg, XP_U16 resID );
|
|
void ceSetChecked( HWND hDlg, XP_U16 resID, XP_Bool check );
|
|
|
|
void ceCenterCtl( HWND hDlg, XP_U16 resID );
|
|
void ceCheckMenus( const CEAppGlobals* globals );
|
|
|
|
void ceGetItemRect( HWND hDlg, XP_U16 resID, RECT* rect );
|
|
void ceMoveItem( HWND hDlg, XP_U16 resID, XP_S16 byX, XP_S16 byY );
|
|
|
|
int ceMessageBoxChar( CEAppGlobals* globals, const XP_UCHAR* str,
|
|
const wchar_t* title, XP_U16 buttons );
|
|
int ceOops( CEAppGlobals* globals, const XP_UCHAR* str );
|
|
|
|
typedef enum {
|
|
PREFS_FILE_PATH_L
|
|
,DEFAULT_DIR_PATH_L
|
|
,DEFAULT_GAME_PATH
|
|
,PROGFILES_PATH
|
|
} CePathType;
|
|
XP_U16 ceGetPath( CEAppGlobals* globals, CePathType typ,
|
|
void* buf, XP_U16 bufLen );
|
|
|
|
/* set vHeight to 0 to turn off scrolling */
|
|
typedef enum { DLG_STATE_NONE = 0
|
|
, DLG_STATE_TRAPBACK = 1
|
|
, DLG_STATE_OKONLY = 2
|
|
, DLG_STATE_DONEONLY = 4
|
|
} DlgStateTask;
|
|
typedef struct CeDlgHdr {
|
|
CEAppGlobals* globals;
|
|
HWND hDlg;
|
|
|
|
/* Below this line is private to ceutil.c */
|
|
DlgStateTask doWhat;
|
|
XP_U16 nPage;
|
|
XP_U16 prevY;
|
|
XP_Bool penDown;
|
|
} CeDlgHdr;
|
|
void ceDlgSetup( CeDlgHdr* dlgHdr, HWND hDlg, DlgStateTask doWhat );
|
|
void ceDlgComboShowHide( CeDlgHdr* dlgHdr, XP_U16 baseId );
|
|
XP_Bool ceDoDlgHandle( CeDlgHdr* dlgHdr, UINT message, WPARAM wParam, LPARAM lParam);
|
|
|
|
/* Are we drawing things in landscape mode? */
|
|
XP_Bool ceIsLandscape( CEAppGlobals* globals );
|
|
|
|
void ceSetLeftSoftkey( CEAppGlobals* globals, XP_U16 id );
|
|
XP_Bool ceGetExeDir( wchar_t* buf, XP_U16 bufLen );
|
|
|
|
#if defined _WIN32_WCE
|
|
void ceSizeIfFullscreen( CEAppGlobals* globals, HWND hWnd );
|
|
#else
|
|
# define ceSizeIfFullscreen( globals, hWnd )
|
|
#endif
|
|
|
|
#ifdef OVERRIDE_BACKKEY
|
|
void trapBackspaceKey( HWND hDlg );
|
|
#else
|
|
# define trapBackspaceKey( hDlg )
|
|
#endif
|
|
|
|
|
|
#endif
|