mirror of
https://github.com/gwenhael-le-moine/x49gp.git
synced 2024-12-26 21:58:41 +01:00
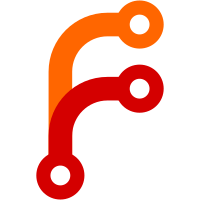
Misc changes, mostly fixes: - fix ./newconfig systems other than OSX (broke inc8b823f
) - fix palette usage in 2-bit color mode (was broken ever since grayscale was implemented in18e1003
and its improperly attributed copyf7913eb
) - fix continuing from breakpoints in the debugger (never worked, was exposed when the debugger was enabled in9c1f2ed
) - restore the printf statements commented out in9c1f2ed
and hide them with #ifdefs instead - close the server socket after accepting a debugger connection to allow another simultaneous debug session to be started using the same TCP port - use the symbolic constant DEFAULT_GDBSTUB_PORT (already defined in gdb_stub.h as 1234) when starting the gdb server in main.c in place of the raw number 1234 - change Makefile to read the name of the firmware file from the file update.scp instead of hardcoding it; this allows users to switch to another firmware by simply pasting it along with its accompanying update.scp into the x49gp directory - Enhance port G (keyboard) handling to remember the value of output bits across periods with these bits configured as input This fixes interaction with HPGCC3 keyboard routines, and it also fixes keys with eint==7 (assuming the stock firmware is in use) needing a double-tap to work unless pressed very shortly after another keypress (the latter broke inb5f93ed
) - Get rid of the deprecated function warning by switching from gdk_pixbuf_new_from_inline to gdk_pixbuf_new_from_data (based on code by chwdt) - Delete remaining now-redundant CVS files - Don't release all buttons anymore if there are still physical keys and/or the left mouse button are holding some down On the other hand, forcibly release all buttons when losing focus to avoid getting stuck in a state with buttons down when they are not held down by anything; this would happen due to missed events while not in focus - Add a context menu to the screen, containing only "Reset" and "Quit" items for now - Ensure that the files backing flash, sram, and s3c2410-sram exist and have the correct size when opening them Note that if the flash file does not exist, this will not fill it with the code that's supposed to be in there, obviously causing the calculator to crash. That's an improvement for later. - Allow the config system to fill not only numbers, but also strings (including filenames) with default values basename is excluded, but it's planned to be dropped entirely. - Add an "install" target to the Makefile - Implement a more generic command-line parser for substantially improved flexibility - Also adds a proper help option, though the manual referenced in the corresponding output (a manpage, hopefully) does not exist yet. - Drop the "basename" config property in favor of interpreting relative paths in the config as relative to the config file's location - Retire the "image" config property in favor of simply loading the image from next to the binary or from the install directory - Split the UI name property into name (affecting only the window title) and type (affecting the UI image and in the future also the default bootcode) properties - Change the default calculator type to the 50g everywhere, which probably matches today's user expectations better than the 49g+. - Create a flash file from the calculator model's appropriate boot file if it does not exist, relying on the bootcode to detect the absence of a firmware The bootcode will complain about the missing firmware and enter update mode, so the user needs to supply their favorite firmware version and point the bootcode's updater to it. The easiest way is probably pointing the emulated SD card at a directory containing the firmware and its accompanying update.scp file, and then starting the SD-based update. - Add SD mount / unmount options to the right-click / menu-key popup menu - Remove most of the old script-based config-generating system since the binary now has these capabilities as well - Add an applications menu item for installing - Keep some debug output on stderr and a huge vvfat.log file from showing up when not debugging x49gp itself - Allow (re-)connecting a debugger to a running session This is done through the right-click / menu-key popup menu. To avoid confusion due to the accidental clicks leading to an unresponsive interface (caused by waiting for the debugger to connect), this option is hidden unless option -d or its new companion -D (same as -d, but does not start the debug interface right away) is present. - Improved support for hardware keyboards - Update README.md, add manpage, rename other README files to TODO to reflect their contents
348 lines
12 KiB
C
348 lines
12 KiB
C
/* $Id: s3c2410.h,v 1.18 2008/12/11 12:18:17 ecd Exp $
|
|
*/
|
|
|
|
#ifndef _X49GP_S3C2410_H
|
|
#define _X49GP_S3C2410_H 1
|
|
|
|
#include <x49gp_types.h>
|
|
|
|
typedef struct {
|
|
const char *name;
|
|
uint32_t reset;
|
|
uint32_t *datap;
|
|
} s3c2410_offset_t;
|
|
|
|
#define S3C2410_OFFSET(module, name, reset, data) \
|
|
[S3C2410_ ## module ## _ ## name >> 2] = { #name, reset, &(data) }
|
|
|
|
#define S3C2410_OFFSET_OK(p, offset) \
|
|
((((offset) >> 2) < (p)->nr_regs) && (p)->regs[(offset) >> 2].name)
|
|
|
|
#define S3C2410_OFFSET_ENTRY(p, offset) &((p)->regs[(offset) >> 2])
|
|
|
|
|
|
#define S3C2410_MAP_SIZE 0x00010000
|
|
|
|
|
|
#define S3C2410_SRAM_BASE 0x40000000
|
|
#define S3C2410_SRAM_SIZE 0x00001000
|
|
|
|
#define S3C2410_MEMC_BASE 0x48000000
|
|
|
|
#define S3C2410_MEMC_BWSCON 0x0000
|
|
#define S3C2410_MEMC_BANKCON0 0x0004
|
|
#define S3C2410_MEMC_BANKCON1 0x0008
|
|
#define S3C2410_MEMC_BANKCON2 0x000c
|
|
#define S3C2410_MEMC_BANKCON3 0x0010
|
|
#define S3C2410_MEMC_BANKCON4 0x0014
|
|
#define S3C2410_MEMC_BANKCON5 0x0018
|
|
#define S3C2410_MEMC_BANKCON6 0x001c
|
|
#define S3C2410_MEMC_BANKCON7 0x0020
|
|
#define S3C2410_MEMC_REFRESH 0x0024
|
|
#define S3C2410_MEMC_BANKSIZE 0x0028
|
|
#define S3C2410_MEMC_MRSRB6 0x002c
|
|
#define S3C2410_MEMC_MRSRB7 0x0030
|
|
|
|
#define S3C2410_USBHOST_BASE 0x49000000
|
|
|
|
#define S3C2410_INTC_BASE 0x4a000000
|
|
|
|
#define S3C2410_INTC_SRCPND 0x0000
|
|
#define S3C2410_INTC_INTMOD 0x0004
|
|
#define S3C2410_INTC_INTMSK 0x0008
|
|
#define S3C2410_INTC_PRIORITY 0x000c
|
|
#define S3C2410_INTC_INTPND 0x0010
|
|
#define S3C2410_INTC_INTOFFSET 0x0014
|
|
#define S3C2410_INTC_SUBSRCPND 0x0018
|
|
#define S3C2410_INTC_INTSUBMSK 0x001c
|
|
|
|
#define S3C2410_POWER_BASE 0x4c000000
|
|
|
|
#define S3C2410_POWER_LOCKTIME 0x0000
|
|
#define S3C2410_POWER_MPLLCON 0x0004
|
|
#define S3C2410_POWER_UPLLCON 0x0008
|
|
#define S3C2410_POWER_CLKCON 0x000c
|
|
#define S3C2410_POWER_CLKSLOW 0x0010
|
|
#define S3C2410_POWER_CLKDIVN 0x0014
|
|
|
|
#define S3C2410_LCD_BASE 0x4d000000
|
|
|
|
#define S3C2410_LCD_LCDCON1 0x0000
|
|
#define S3C2410_LCD_LCDCON2 0x0004
|
|
#define S3C2410_LCD_LCDCON3 0x0008
|
|
#define S3C2410_LCD_LCDCON4 0x000c
|
|
#define S3C2410_LCD_LCDCON5 0x0010
|
|
#define S3C2410_LCD_LCDSADDR1 0x0014
|
|
#define S3C2410_LCD_LCDSADDR2 0x0018
|
|
#define S3C2410_LCD_LCDSADDR3 0x001c
|
|
#define S3C2410_LCD_REDLUT 0x0020
|
|
#define S3C2410_LCD_GREENLUT 0x0024
|
|
#define S3C2410_LCD_BLUELUT 0x0028
|
|
#define S3C2410_LCD_DITHMODE 0x004c
|
|
#define S3C2410_LCD_TPAL 0x0050
|
|
#define S3C2410_LCD_LCDINTPND 0x0054
|
|
#define S3C2410_LCD_LCDSRCPND 0x0058
|
|
#define S3C2410_LCD_LCDINTMSK 0x005c
|
|
#define S3C2410_LCD_LPCSEL 0x0060
|
|
#define S3C2410_LCD_UNKNOWN_68 0x0068
|
|
|
|
#define S3C2410_LCD_PALETTE_START 0x0400
|
|
#define S3C2410_LCD_PALETTE_SIZE 0x0400
|
|
|
|
#define S3C2410_NAND_BASE 0x4e000000
|
|
|
|
#define S3C2410_NAND_NFCONF 0x0000
|
|
#define S3C2410_NAND_NFCMD 0x0004
|
|
#define S3C2410_NAND_NFADDR 0x0008
|
|
#define S3C2410_NAND_NFDATA 0x000c
|
|
#define S3C2410_NAND_NFSTAT 0x0010
|
|
#define S3C2410_NAND_NFECC 0x0014
|
|
|
|
#define S3C2410_UART0_BASE 0x50000000
|
|
#define S3C2410_UART1_BASE 0x50004000
|
|
#define S3C2410_UART2_BASE 0x50008000
|
|
|
|
#define S3C2410_UART0_ULCON 0x0000
|
|
#define S3C2410_UART0_UCON 0x0004
|
|
#define S3C2410_UART0_UFCON 0x0008
|
|
#define S3C2410_UART0_UMCON 0x000c
|
|
#define S3C2410_UART0_UTRSTAT 0x0010
|
|
#define S3C2410_UART0_UERSTAT 0x0014
|
|
#define S3C2410_UART0_UFSTAT 0x0018
|
|
#define S3C2410_UART0_UMSTAT 0x001c
|
|
#define S3C2410_UART0_UTXH 0x0020
|
|
#define S3C2410_UART0_URXH 0x0024
|
|
#define S3C2410_UART0_UBRDIV 0x0028
|
|
|
|
#define S3C2410_UART1_ULCON 0x0000
|
|
#define S3C2410_UART1_UCON 0x0004
|
|
#define S3C2410_UART1_UFCON 0x0008
|
|
#define S3C2410_UART1_UMCON 0x000c
|
|
#define S3C2410_UART1_UTRSTAT 0x0010
|
|
#define S3C2410_UART1_UERSTAT 0x0014
|
|
#define S3C2410_UART1_UFSTAT 0x0018
|
|
#define S3C2410_UART1_UMSTAT 0x001c
|
|
#define S3C2410_UART1_UTXH 0x0020
|
|
#define S3C2410_UART1_URXH 0x0024
|
|
#define S3C2410_UART1_UBRDIV 0x0028
|
|
|
|
#define S3C2410_UART2_ULCON 0x0000
|
|
#define S3C2410_UART2_UCON 0x0004
|
|
#define S3C2410_UART2_UFCON 0x0008
|
|
#define S3C2410_UART2_UTRSTAT 0x0010
|
|
#define S3C2410_UART2_UERSTAT 0x0014
|
|
#define S3C2410_UART2_UFSTAT 0x0018
|
|
#define S3C2410_UART2_UTXH 0x0020
|
|
#define S3C2410_UART2_URXH 0x0024
|
|
#define S3C2410_UART2_UBRDIV 0x0028
|
|
|
|
#define S3C2410_TIMER_BASE 0x51000000
|
|
|
|
#define S3C2410_TIMER_TCFG0 0x0000
|
|
#define S3C2410_TIMER_TCFG1 0x0004
|
|
#define S3C2410_TIMER_TCON 0x0008
|
|
#define S3C2410_TIMER_TCNTB0 0x000c
|
|
#define S3C2410_TIMER_TCMPB0 0x0010
|
|
#define S3C2410_TIMER_TCNTO0 0x0014
|
|
#define S3C2410_TIMER_TCNTB1 0x0018
|
|
#define S3C2410_TIMER_TCMPB1 0x001c
|
|
#define S3C2410_TIMER_TCNTO1 0x0020
|
|
#define S3C2410_TIMER_TCNTB2 0x0024
|
|
#define S3C2410_TIMER_TCMPB2 0x0028
|
|
#define S3C2410_TIMER_TCNTO2 0x002c
|
|
#define S3C2410_TIMER_TCNTB3 0x0030
|
|
#define S3C2410_TIMER_TCMPB3 0x0034
|
|
#define S3C2410_TIMER_TCNTO3 0x0038
|
|
#define S3C2410_TIMER_TCNTB4 0x003c
|
|
#define S3C2410_TIMER_TCNTO4 0x0040
|
|
|
|
#define S3C2410_USBDEV_BASE 0x52000000
|
|
|
|
#define S3C2410_USBDEV_FUNC_ADDR_REG 0x0140
|
|
#define S3C2410_USBDEV_PWR_REG 0x0144
|
|
#define S3C2410_USBDEV_EP_INT_REG 0x0148
|
|
#define S3C2410_USBDEV_USB_INT_REG 0x0158
|
|
#define S3C2410_USBDEV_EP_INT_EN_REG 0x015c
|
|
#define S3C2410_USBDEV_USB_INT_EN_REG 0x016c
|
|
#define S3C2410_USBDEV_FRAME_NUM1_REG 0x0170
|
|
#define S3C2410_USBDEV_FRAME_NUM2_REG 0x0174
|
|
#define S3C2410_USBDEV_INDEX_REG 0x0178
|
|
#define S3C2410_USBDEV_EP0_FIFO_REG 0x01C0
|
|
#define S3C2410_USBDEV_EP1_FIFO_REG 0x01C4
|
|
#define S3C2410_USBDEV_EP2_FIFO_REG 0x01C8
|
|
#define S3C2410_USBDEV_EP3_FIFO_REG 0x01CC
|
|
#define S3C2410_USBDEV_EP4_FIFO_REG 0x01D0
|
|
#define S3C2410_USBDEV_EP1_DMA_CON 0x0200
|
|
#define S3C2410_USBDEV_EP1_DMA_UNIT 0x0204
|
|
#define S3C2410_USBDEV_EP1_DMA_FIFO 0x0208
|
|
#define S3C2410_USBDEV_EP1_DMA_TTC_L 0x020C
|
|
#define S3C2410_USBDEV_EP1_DMA_TTC_M 0x0210
|
|
#define S3C2410_USBDEV_EP1_DMA_TTC_H 0x0214
|
|
#define S3C2410_USBDEV_EP2_DMA_CON 0x0218
|
|
#define S3C2410_USBDEV_EP2_DMA_UNIT 0x021C
|
|
#define S3C2410_USBDEV_EP2_DMA_FIFO 0x0220
|
|
#define S3C2410_USBDEV_EP2_DMA_TTC_L 0x0224
|
|
#define S3C2410_USBDEV_EP2_DMA_TTC_M 0x0228
|
|
#define S3C2410_USBDEV_EP2_DMA_TTC_H 0x022C
|
|
#define S3C2410_USBDEV_EP3_DMA_CON 0x0240
|
|
#define S3C2410_USBDEV_EP3_DMA_UNIT 0x0244
|
|
#define S3C2410_USBDEV_EP3_DMA_FIFO 0x0248
|
|
#define S3C2410_USBDEV_EP3_DMA_TTC_L 0x024C
|
|
#define S3C2410_USBDEV_EP3_DMA_TTC_M 0x0250
|
|
#define S3C2410_USBDEV_EP3_DMA_TTC_H 0x0254
|
|
#define S3C2410_USBDEV_EP4_DMA_CON 0x0258
|
|
#define S3C2410_USBDEV_EP4_DMA_UNIT 0x025C
|
|
#define S3C2410_USBDEV_EP4_DMA_FIFO 0x0260
|
|
#define S3C2410_USBDEV_EP4_DMA_TTC_L 0x0264
|
|
#define S3C2410_USBDEV_EP4_DMA_TTC_M 0x0268
|
|
#define S3C2410_USBDEV_EP4_DMA_TTC_H 0x026C
|
|
#define S3C2410_USBDEV_MAXP_REG_WRONG 0x0180
|
|
#define S3C2410_USBDEV_IN_CSR1_REG_EP0_CSR 0x0184
|
|
#define S3C2410_USBDEV_IN_CSR2_REG 0x0188
|
|
#define S3C2410_USBDEV_MAXP_REG 0x018c
|
|
#define S3C2410_USBDEV_OUT_CSR1_REG 0x0190
|
|
#define S3C2410_USBDEV_OUT_CSR2_REG 0x0194
|
|
#define S3C2410_USBDEV_OUT_FIFO_CNT1_REG 0x0198
|
|
#define S3C2410_USBDEV_OUT_FIFO_CNT2_REG 0x019C
|
|
|
|
#define S3C2410_WATCHDOG_BASE 0x53000000
|
|
|
|
#define S3C2410_WATCHDOG_WTCON 0x0000
|
|
#define S3C2410_WATCHDOG_WTDAT 0x0004
|
|
#define S3C2410_WATCHDOG_WTCNT 0x0008
|
|
|
|
#define S3C2410_IO_PORT_BASE 0x56000000
|
|
|
|
#define S3C2410_IO_PORT_GPACON 0x0000
|
|
#define S3C2410_IO_PORT_GPADAT 0x0004
|
|
#define S3C2410_IO_PORT_GPBCON 0x0010
|
|
#define S3C2410_IO_PORT_GPBDAT 0x0014
|
|
#define S3C2410_IO_PORT_GPBUP 0x0018
|
|
#define S3C2410_IO_PORT_GPCCON 0x0020
|
|
#define S3C2410_IO_PORT_GPCDAT 0x0024
|
|
#define S3C2410_IO_PORT_GPCUP 0x0028
|
|
#define S3C2410_IO_PORT_GPDCON 0x0030
|
|
#define S3C2410_IO_PORT_GPDDAT 0x0034
|
|
#define S3C2410_IO_PORT_GPDUP 0x0038
|
|
#define S3C2410_IO_PORT_GPECON 0x0040
|
|
#define S3C2410_IO_PORT_GPEDAT 0x0044
|
|
#define S3C2410_IO_PORT_GPEUP 0x0048
|
|
#define S3C2410_IO_PORT_GPFCON 0x0050
|
|
#define S3C2410_IO_PORT_GPFDAT 0x0054
|
|
#define S3C2410_IO_PORT_GPFUP 0x0058
|
|
#define S3C2410_IO_PORT_GPGCON 0x0060
|
|
#define S3C2410_IO_PORT_GPGDAT 0x0064
|
|
#define S3C2410_IO_PORT_GPGUP 0x0068
|
|
#define S3C2410_IO_PORT_GPHCON 0x0070
|
|
#define S3C2410_IO_PORT_GPHDAT 0x0074
|
|
#define S3C2410_IO_PORT_GPHUP 0x0078
|
|
#define S3C2410_IO_PORT_MISCCR 0x0080
|
|
#define S3C2410_IO_PORT_DCLKCON 0x0084
|
|
#define S3C2410_IO_PORT_EXTINT0 0x0088
|
|
#define S3C2410_IO_PORT_EXTINT1 0x008c
|
|
#define S3C2410_IO_PORT_EXTINT2 0x0090
|
|
#define S3C2410_IO_PORT_EINTFLT0 0x0094
|
|
#define S3C2410_IO_PORT_EINTFLT1 0x0098
|
|
#define S3C2410_IO_PORT_EINTFLT2 0x009c
|
|
#define S3C2410_IO_PORT_EINTFLT3 0x00a0
|
|
#define S3C2410_IO_PORT_EINTMASK 0x00a4
|
|
#define S3C2410_IO_PORT_EINTPEND 0x00a8
|
|
#define S3C2410_IO_PORT_GSTATUS0 0x00ac
|
|
#define S3C2410_IO_PORT_GSTATUS1 0x00b0
|
|
#define S3C2410_IO_PORT_GSTATUS2 0x00b4
|
|
#define S3C2410_IO_PORT_GSTATUS3 0x00b8
|
|
#define S3C2410_IO_PORT_GSTATUS4 0x00bc
|
|
|
|
#define S3C2410_RTC_BASE 0x57000000
|
|
|
|
#define S3C2410_RTC_RTCCON 0x0040
|
|
#define S3C2410_RTC_TICNT 0x0044
|
|
#define S3C2410_RTC_RTCALM 0x0050
|
|
#define S3C2410_RTC_ALMSEC 0x0054
|
|
#define S3C2410_RTC_ALMMIN 0x0058
|
|
#define S3C2410_RTC_ALMHOUR 0x005c
|
|
#define S3C2410_RTC_ALMDATE 0x0060
|
|
#define S3C2410_RTC_ALMMON 0x0064
|
|
#define S3C2410_RTC_ALMYEAR 0x0068
|
|
#define S3C2410_RTC_RTCRST 0x006c
|
|
#define S3C2410_RTC_BCDSEC 0x0070
|
|
#define S3C2410_RTC_BCDMIN 0x0074
|
|
#define S3C2410_RTC_BCDHOUR 0x0078
|
|
#define S3C2410_RTC_BCDDATE 0x007c
|
|
#define S3C2410_RTC_BCDDAY 0x0080
|
|
#define S3C2410_RTC_BCDMON 0x0084
|
|
#define S3C2410_RTC_BCDYEAR 0x0088
|
|
|
|
#define S3C2410_ADC_BASE 0x58000000
|
|
|
|
#define S3C2410_ADC_ADCCON 0x0000
|
|
#define S3C2410_ADC_ADCTSC 0x0004
|
|
#define S3C2410_ADC_ADCDLY 0x0008
|
|
#define S3C2410_ADC_ADCDAT0 0x000c
|
|
#define S3C2410_ADC_ADCDAT1 0x0010
|
|
|
|
#define S3C2410_SPI_BASE 0x59000000
|
|
|
|
#define S3C2410_SPI_SPICON0 0x0000
|
|
#define S3C2410_SPI_SPISTA0 0x0004
|
|
#define S3C2410_SPI_SPPIN0 0x0008
|
|
#define S3C2410_SPI_SPPRE0 0x000c
|
|
#define S3C2410_SPI_SPTDAT0 0x0010
|
|
#define S3C2410_SPI_SPRDAT0 0x0014
|
|
#define S3C2410_SPI_SPICON1 0x0020
|
|
#define S3C2410_SPI_SPISTA1 0x0024
|
|
#define S3C2410_SPI_SPPIN1 0x0028
|
|
#define S3C2410_SPI_SPPRE1 0x002c
|
|
#define S3C2410_SPI_SPTDAT1 0x0030
|
|
#define S3C2410_SPI_SPRDAT1 0x0034
|
|
|
|
#define S3C2410_SDI_BASE 0x5a000000
|
|
|
|
#define S3C2410_SDI_SDICON 0x0000
|
|
#define S3C2410_SDI_SDIPRE 0x0004
|
|
#define S3C2410_SDI_SDICARG 0x0008
|
|
#define S3C2410_SDI_SDICCON 0x000c
|
|
#define S3C2410_SDI_SDICSTA 0x0010
|
|
#define S3C2410_SDI_SDIRSP0 0x0014
|
|
#define S3C2410_SDI_SDIRSP1 0x0018
|
|
#define S3C2410_SDI_SDIRSP2 0x001c
|
|
#define S3C2410_SDI_SDIRSP3 0x0020
|
|
#define S3C2410_SDI_SDIDTIMER 0x0024
|
|
#define S3C2410_SDI_SDIBSIZE 0x0028
|
|
#define S3C2410_SDI_SDIDCON 0x002c
|
|
#define S3C2410_SDI_SDIDCNT 0x0030
|
|
#define S3C2410_SDI_SDIDSTA 0x0034
|
|
#define S3C2410_SDI_SDIFSTA 0x0038
|
|
#define S3C2410_SDI_SDIDAT 0x003c
|
|
#define S3C2410_SDI_SDIIMSK 0x0040
|
|
|
|
|
|
extern int x49gp_s3c2410_init(x49gp_t *x49gp);
|
|
extern int x49gp_s3c2410_arm_init(x49gp_t *x49gp);
|
|
extern int x49gp_s3c2410_mmu_init(x49gp_t *x49gp);
|
|
extern int x49gp_s3c2410_sram_init(x49gp_t *x49gp);
|
|
extern int x49gp_s3c2410_memc_init(x49gp_t *x49gp);
|
|
extern int x49gp_s3c2410_intc_init(x49gp_t *x49gp);
|
|
extern int x49gp_s3c2410_power_init(x49gp_t *x49gp);
|
|
extern int x49gp_s3c2410_lcd_init(x49gp_t *x49gp);
|
|
extern int x49gp_s3c2410_nand_init(x49gp_t *x49gp);
|
|
extern int x49gp_s3c2410_uart_init(x49gp_t *x49gp);
|
|
extern int x49gp_s3c2410_timer_init(x49gp_t *x49gp);
|
|
extern int x49gp_s3c2410_usbdev_init(x49gp_t *x49gp);
|
|
extern int x49gp_s3c2410_watchdog_init(x49gp_t *x49gp);
|
|
extern int x49gp_s3c2410_io_port_init(x49gp_t *x49gp);
|
|
extern int x49gp_s3c2410_rtc_init(x49gp_t *x49gp);
|
|
extern int x49gp_s3c2410_adc_init(x49gp_t *x49gp);
|
|
extern int x49gp_s3c2410_spi_init(x49gp_t *x49gp);
|
|
extern int x49gp_s3c2410_sdi_init(x49gp_t *x49gp);
|
|
|
|
extern void s3c2410_io_port_g_update(x49gp_t *x49gp, int column, int row, unsigned char columnbit, unsigned char rowbit, uint32_t new_state);
|
|
extern void s3c2410_io_port_f_set_bit(x49gp_t *x49gp, int n, uint32_t set);
|
|
|
|
extern void x49gp_schedule_lcd_update(x49gp_t *x49gp);
|
|
extern void x49gp_lcd_update(x49gp_t *x49gp);
|
|
|
|
extern unsigned long s3c2410_timer_next_interrupt(x49gp_t *x49gp);
|
|
extern unsigned long s3c2410_watchdog_next_interrupt(x49gp_t *x49gp);
|
|
|
|
#endif /* !(_X49GP_S3C2410_H) */
|