mirror of
https://github.com/gwenhael-le-moine/sway-patched-tray-menu.git
synced 2024-12-27 21:58:16 +01:00
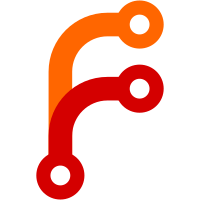
This allows for an optional validation stage when storing an input config. Currently, only the xkb keymap is validated. If storing the delta input config will result in any invalid xkb keymaps, the input config will not be stored and error will be populated with the first line of the xkbcommon log.
113 lines
3.3 KiB
C
113 lines
3.3 KiB
C
#include <string.h>
|
|
#include <strings.h>
|
|
#include "sway/commands.h"
|
|
#include "sway/input/input-manager.h"
|
|
#include "sway/input/keyboard.h"
|
|
#include "log.h"
|
|
#include "stringop.h"
|
|
|
|
// must be in order for the bsearch
|
|
static struct cmd_handler input_handlers[] = {
|
|
{ "accel_profile", input_cmd_accel_profile },
|
|
{ "click_method", input_cmd_click_method },
|
|
{ "drag", input_cmd_drag },
|
|
{ "drag_lock", input_cmd_drag_lock },
|
|
{ "dwt", input_cmd_dwt },
|
|
{ "events", input_cmd_events },
|
|
{ "left_handed", input_cmd_left_handed },
|
|
{ "map_from_region", input_cmd_map_from_region },
|
|
{ "map_to_output", input_cmd_map_to_output },
|
|
{ "middle_emulation", input_cmd_middle_emulation },
|
|
{ "natural_scroll", input_cmd_natural_scroll },
|
|
{ "pointer_accel", input_cmd_pointer_accel },
|
|
{ "repeat_delay", input_cmd_repeat_delay },
|
|
{ "repeat_rate", input_cmd_repeat_rate },
|
|
{ "scroll_button", input_cmd_scroll_button },
|
|
{ "scroll_factor", input_cmd_scroll_factor },
|
|
{ "scroll_method", input_cmd_scroll_method },
|
|
{ "tap", input_cmd_tap },
|
|
{ "tap_button_map", input_cmd_tap_button_map },
|
|
{ "xkb_layout", input_cmd_xkb_layout },
|
|
{ "xkb_model", input_cmd_xkb_model },
|
|
{ "xkb_options", input_cmd_xkb_options },
|
|
{ "xkb_rules", input_cmd_xkb_rules },
|
|
{ "xkb_variant", input_cmd_xkb_variant },
|
|
};
|
|
|
|
// must be in order for the bsearch
|
|
static struct cmd_handler input_config_handlers[] = {
|
|
{ "xkb_capslock", input_cmd_xkb_capslock },
|
|
{ "xkb_numlock", input_cmd_xkb_numlock },
|
|
};
|
|
|
|
/**
|
|
* Re-translate keysyms if a change in the input config could affect them.
|
|
*/
|
|
static void retranslate_keysyms(struct input_config *input_config) {
|
|
for (int i = 0; i < config->input_configs->length; ++i) {
|
|
struct input_config *ic = config->input_configs->items[i];
|
|
if (ic->xkb_layout) {
|
|
// this is the first config with xkb_layout
|
|
if (ic->identifier == input_config->identifier) {
|
|
translate_keysyms(ic);
|
|
}
|
|
|
|
return;
|
|
}
|
|
}
|
|
}
|
|
|
|
struct cmd_results *cmd_input(int argc, char **argv) {
|
|
struct cmd_results *error = NULL;
|
|
if ((error = checkarg(argc, "input", EXPECTED_AT_LEAST, 2))) {
|
|
return error;
|
|
}
|
|
|
|
sway_log(SWAY_DEBUG, "entering input block: %s", argv[0]);
|
|
|
|
config->handler_context.input_config = new_input_config(argv[0]);
|
|
if (!config->handler_context.input_config) {
|
|
return cmd_results_new(CMD_FAILURE, "Couldn't allocate config");
|
|
}
|
|
|
|
struct cmd_results *res;
|
|
|
|
if (find_handler(argv[1], input_config_handlers,
|
|
sizeof(input_config_handlers))) {
|
|
if (config->reading) {
|
|
res = config_subcommand(argv + 1, argc - 1,
|
|
input_config_handlers, sizeof(input_config_handlers));
|
|
} else {
|
|
res = cmd_results_new(CMD_FAILURE,
|
|
"Can only be used in config file.");
|
|
}
|
|
} else {
|
|
res = config_subcommand(argv + 1, argc - 1,
|
|
input_handlers, sizeof(input_handlers));
|
|
}
|
|
|
|
if (!res || res->status == CMD_SUCCESS) {
|
|
char *error = NULL;
|
|
struct input_config *ic =
|
|
store_input_config(config->handler_context.input_config, &error);
|
|
if (!ic) {
|
|
free_input_config(config->handler_context.input_config);
|
|
if (res) {
|
|
free_cmd_results(res);
|
|
}
|
|
res = cmd_results_new(CMD_FAILURE, "Failed to compile keymap: %s",
|
|
error ? error : "(details unavailable)");
|
|
free(error);
|
|
return res;
|
|
}
|
|
|
|
input_manager_apply_input_config(ic);
|
|
retranslate_keysyms(ic);
|
|
} else {
|
|
free_input_config(config->handler_context.input_config);
|
|
}
|
|
|
|
config->handler_context.input_config = NULL;
|
|
|
|
return res;
|
|
}
|