mirror of
https://github.com/mamedev/mame.git
synced 2024-11-16 07:48:32 +01:00
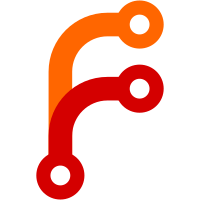
* Moved several machine lifecycle callbacks to the notifier/subscriber model. The old callback registration model is still available for them for now, but prints a deprecation warning. * Added pre-save/post-load notifications. * Use a single allocated timer rather than one anonymous timer per waiter. Waiters no longer prevent saved states from being loaded. * Clean up outstanding waiters on stop or state load rather than just leaking them. * Started documenting parts of the emulator interface object that should be relatively stable. -imagedev/avivideo.cpp: Fixed an object leak on unload. Also changed some other media image devices to use smart pointers.
79 lines
2.3 KiB
Lua
79 lines
2.3 KiB
Lua
-- license:BSD-3-Clause
|
|
-- copyright-holders:Vas Crabb
|
|
-- TODO: track time properly across soft reset and state load
|
|
local exports = {
|
|
name = 'timer',
|
|
version = '0.0.3',
|
|
description = 'Game play timer',
|
|
license = 'BSD-3-Clause',
|
|
author = { name = 'Vas Crabb' } }
|
|
|
|
local timer = exports
|
|
|
|
local reset_subscription, stop_subscription
|
|
|
|
function timer.startplugin()
|
|
local total_time = 0
|
|
local start_time = 0
|
|
local play_count = 0
|
|
local emu_total = emu.attotime()
|
|
|
|
local reference = 0
|
|
local lastupdate
|
|
local highlight -- hacky - workaround for the menu not remembering the selected item if its ref is nullptr
|
|
|
|
|
|
local function sectohms(time)
|
|
local hrs = time // 3600
|
|
local min = (time % 3600) // 60
|
|
local sec = time % 60
|
|
return string.format(_p('plugin-timer', '%03d:%02d:%02d'), hrs, min, sec)
|
|
end
|
|
|
|
local function menu_populate()
|
|
lastupdate = os.time()
|
|
local refname = (reference == 0) and _p('plugin-timer', 'Wall clock') or _p('plugin-timer', 'Emulated time')
|
|
local time = (reference == 0) and (lastupdate - start_time) or manager.machine.time.seconds
|
|
local total = (reference == 0) and (total_time + time) or (manager.machine.time + emu_total).seconds
|
|
return
|
|
{
|
|
{ _p("plugin-timer", "Reference"), refname, (reference == 0) and 'r' or 'l' },
|
|
{ '---', '', '' },
|
|
{ _p("plugin-timer", "Current time"), sectohms(time), "off" },
|
|
{ _p("plugin-timer", "Total time"), sectohms(total), "off" },
|
|
{ _p("plugin-timer", "Play Count"), tostring(play_count), "off" } },
|
|
highlight,
|
|
"idle"
|
|
end
|
|
|
|
local function menu_callback(index, event)
|
|
if (index == 1) and ((event == 'left') or (event == 'right') or (event == 'select')) then
|
|
reference = reference ~ 1
|
|
return true
|
|
end
|
|
highlight = index
|
|
return os.time() > lastupdate
|
|
end
|
|
|
|
|
|
reset_subscription = emu.add_machine_reset_notifier(
|
|
function ()
|
|
if emu.romname() ~= '___empty' then
|
|
start_time = os.time()
|
|
local persister = require('timer/timer_persist')
|
|
total_time, play_count, emu_total = persister:start_session()
|
|
end
|
|
end)
|
|
|
|
stop_subscription = emu.add_machine_stop_notifier(
|
|
function ()
|
|
if emu.romname() ~= '___empty' then
|
|
local persister = require('timer/timer_persist')
|
|
persister:update_totals(start_time)
|
|
end
|
|
end)
|
|
|
|
emu.register_menu(menu_callback, menu_populate, _p("plugin-timer", "Timer"))
|
|
end
|
|
|
|
return exports
|