mirror of
https://gitlab.freedesktop.org/emersion/libliftoff.git
synced 2024-11-16 19:47:55 +01:00
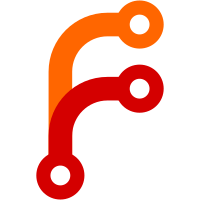
This lets the library user define a layer where composition will happen. At the moment there can be at most one composition layer per output, on the primary plane. It should be possible to remove these restrictions in the future if desirable. The composition layer will be put on a plane if and only if composition is needed. The compositor needs to know where composited layers will be blended to resolve zpos conflicts. Closes: https://github.com/emersion/libliftoff/issues/9
48 lines
1 KiB
C
48 lines
1 KiB
C
#include <assert.h>
|
|
#include <stdlib.h>
|
|
#include <string.h>
|
|
#include <sys/types.h>
|
|
#include "private.h"
|
|
|
|
struct liftoff_output *liftoff_output_create(struct liftoff_display *display,
|
|
uint32_t crtc_id)
|
|
{
|
|
struct liftoff_output *output;
|
|
ssize_t crtc_index;
|
|
size_t i;
|
|
|
|
crtc_index = -1;
|
|
for (i = 0; i < display->crtcs_len; i++) {
|
|
if (display->crtcs[i] == crtc_id) {
|
|
crtc_index = i;
|
|
break;
|
|
}
|
|
}
|
|
if (crtc_index < 0) {
|
|
return NULL;
|
|
}
|
|
|
|
output = calloc(1, sizeof(*output));
|
|
if (output == NULL) {
|
|
return NULL;
|
|
}
|
|
output->display = display;
|
|
output->crtc_id = crtc_id;
|
|
output->crtc_index = crtc_index;
|
|
liftoff_list_init(&output->layers);
|
|
liftoff_list_insert(&display->outputs, &output->link);
|
|
return output;
|
|
}
|
|
|
|
void liftoff_output_destroy(struct liftoff_output *output)
|
|
{
|
|
liftoff_list_remove(&output->link);
|
|
free(output);
|
|
}
|
|
|
|
void liftoff_output_set_composition_layer(struct liftoff_output *output,
|
|
struct liftoff_layer *layer)
|
|
{
|
|
assert(layer->output == output);
|
|
output->composition_layer = layer;
|
|
}
|