mirror of
https://gitlab.freedesktop.org/emersion/libliftoff.git
synced 2024-12-27 21:59:24 +01:00
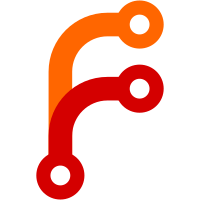
This lets the library user define a layer where composition will happen. At the moment there can be at most one composition layer per output, on the primary plane. It should be possible to remove these restrictions in the future if desirable. The composition layer will be put on a plane if and only if composition is needed. The compositor needs to know where composited layers will be blended to resolve zpos conflicts. Closes: https://github.com/emersion/libliftoff/issues/9
73 lines
1.5 KiB
C
73 lines
1.5 KiB
C
#ifndef PRIVATE_H
|
|
#define PRIVATE_H
|
|
|
|
#include <libliftoff.h>
|
|
#include "list.h"
|
|
#include "log.h"
|
|
|
|
struct liftoff_display {
|
|
int drm_fd;
|
|
|
|
struct liftoff_list planes; /* liftoff_plane.link */
|
|
struct liftoff_list outputs; /* liftoff_output.link */
|
|
|
|
uint32_t *crtcs;
|
|
size_t crtcs_len;
|
|
};
|
|
|
|
struct liftoff_output {
|
|
struct liftoff_display *display;
|
|
uint32_t crtc_id;
|
|
size_t crtc_index;
|
|
struct liftoff_list link; /* liftoff_display.outputs */
|
|
|
|
struct liftoff_layer *composition_layer;
|
|
|
|
struct liftoff_list layers; /* liftoff_layer.link */
|
|
};
|
|
|
|
struct liftoff_layer {
|
|
struct liftoff_output *output;
|
|
struct liftoff_list link; /* liftoff_output.layers */
|
|
|
|
struct liftoff_layer_property *props;
|
|
size_t props_len;
|
|
|
|
struct liftoff_plane *plane;
|
|
};
|
|
|
|
struct liftoff_layer_property {
|
|
char name[DRM_PROP_NAME_LEN];
|
|
uint64_t value;
|
|
};
|
|
|
|
struct liftoff_plane {
|
|
uint32_t id;
|
|
uint32_t possible_crtcs;
|
|
uint32_t type;
|
|
int zpos; /* greater values mean closer to the eye */
|
|
/* TODO: formats */
|
|
struct liftoff_list link; /* liftoff_display.planes */
|
|
|
|
struct liftoff_plane_property *props;
|
|
size_t props_len;
|
|
|
|
struct liftoff_layer *layer;
|
|
};
|
|
|
|
struct liftoff_plane_property {
|
|
char name[DRM_PROP_NAME_LEN];
|
|
uint32_t id;
|
|
};
|
|
|
|
struct liftoff_rect {
|
|
int x, y;
|
|
int width, height;
|
|
};
|
|
|
|
struct liftoff_layer_property *layer_get_property(struct liftoff_layer *layer,
|
|
const char *name);
|
|
void layer_get_rect(struct liftoff_layer *layer, struct liftoff_rect *rect);
|
|
bool layer_intersects(struct liftoff_layer *a, struct liftoff_layer *b);
|
|
|
|
#endif
|