mirror of
https://gitlab.freedesktop.org/emersion/libliftoff.git
synced 2024-12-27 21:59:24 +01:00
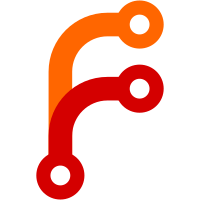
On debug verbosity logging every reuse of planes is spamming the log making it difficult to debug the process. With this patch only the change of reusing the same allocation is logged and how often the allocation was reused after this changed back again.
103 lines
2.6 KiB
C
103 lines
2.6 KiB
C
#ifndef PRIVATE_H
|
|
#define PRIVATE_H
|
|
|
|
#include <libliftoff.h>
|
|
#include "list.h"
|
|
#include "log.h"
|
|
|
|
/* Layer priority is assigned depending on the number of updates during a
|
|
* given number of page-flips */
|
|
#define LIFTOFF_PRIORITY_PERIOD 60
|
|
|
|
struct liftoff_device {
|
|
int drm_fd;
|
|
|
|
struct liftoff_list planes; /* liftoff_plane.link */
|
|
struct liftoff_list outputs; /* liftoff_output.link */
|
|
|
|
uint32_t *crtcs;
|
|
size_t crtcs_len;
|
|
|
|
int page_flip_counter;
|
|
};
|
|
|
|
struct liftoff_output {
|
|
struct liftoff_device *device;
|
|
uint32_t crtc_id;
|
|
size_t crtc_index;
|
|
struct liftoff_list link; /* liftoff_device.outputs */
|
|
|
|
struct liftoff_layer *composition_layer;
|
|
|
|
struct liftoff_list layers; /* liftoff_layer.link */
|
|
/* layer added or removed, or composition layer changed */
|
|
bool layers_changed;
|
|
|
|
int alloc_reused_counter;
|
|
};
|
|
|
|
struct liftoff_layer {
|
|
struct liftoff_output *output;
|
|
struct liftoff_list link; /* liftoff_output.layers */
|
|
|
|
struct liftoff_layer_property *props;
|
|
size_t props_len;
|
|
|
|
bool force_composition; /* FB needs to be composited */
|
|
|
|
struct liftoff_plane *plane;
|
|
|
|
int current_priority, pending_priority;
|
|
};
|
|
|
|
struct liftoff_layer_property {
|
|
char name[DRM_PROP_NAME_LEN];
|
|
uint64_t value;
|
|
bool changed;
|
|
};
|
|
|
|
struct liftoff_plane {
|
|
uint32_t id;
|
|
uint32_t possible_crtcs;
|
|
uint32_t type;
|
|
int zpos; /* greater values mean closer to the eye */
|
|
/* TODO: formats */
|
|
struct liftoff_list link; /* liftoff_device.planes */
|
|
|
|
struct liftoff_plane_property *props;
|
|
size_t props_len;
|
|
|
|
struct liftoff_layer *layer;
|
|
};
|
|
|
|
struct liftoff_plane_property {
|
|
char name[DRM_PROP_NAME_LEN];
|
|
uint32_t id;
|
|
};
|
|
|
|
struct liftoff_rect {
|
|
int x, y;
|
|
int width, height;
|
|
};
|
|
|
|
bool device_test_commit(struct liftoff_device *device,
|
|
drmModeAtomicReq *req, bool *compatible);
|
|
|
|
struct liftoff_layer_property *layer_get_property(struct liftoff_layer *layer,
|
|
const char *name);
|
|
void layer_get_rect(struct liftoff_layer *layer, struct liftoff_rect *rect);
|
|
bool layer_intersects(struct liftoff_layer *a, struct liftoff_layer *b);
|
|
void layer_mark_clean(struct liftoff_layer *layer);
|
|
void layer_update_priority(struct liftoff_layer *layer, bool make_current);
|
|
bool layer_has_fb(struct liftoff_layer *layer);
|
|
|
|
struct liftoff_plane *plane_create(struct liftoff_device *device, uint32_t id);
|
|
void plane_destroy(struct liftoff_plane *plane);
|
|
struct liftoff_plane_property *plane_get_property(struct liftoff_plane *plane,
|
|
const char *name);
|
|
bool plane_apply(struct liftoff_plane *plane, struct liftoff_layer *layer,
|
|
drmModeAtomicReq *req, bool *compatible);
|
|
|
|
void output_log_layers(struct liftoff_output *output);
|
|
|
|
#endif
|