mirror of
https://gitlab.freedesktop.org/emersion/libliftoff.git
synced 2024-11-16 19:47:55 +01:00
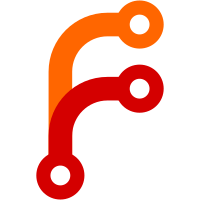
This allows fast access to properties the library cares about. Not very useful yet, but will prove more useful in the future. Closes: https://github.com/emersion/libliftoff/issues/1
99 lines
2.5 KiB
C
99 lines
2.5 KiB
C
#include <stdlib.h>
|
|
#include <string.h>
|
|
#include <unistd.h>
|
|
#include "log.h"
|
|
#include "private.h"
|
|
|
|
ssize_t basic_property_index(const char *name)
|
|
{
|
|
if (strcmp(name, "FB_ID") == 0) {
|
|
return LIFTOFF_PROP_FB_ID;
|
|
} else if (strcmp(name, "CRTC_ID") == 0) {
|
|
return LIFTOFF_PROP_CRTC_ID;
|
|
} else if (strcmp(name, "CRTC_X") == 0) {
|
|
return LIFTOFF_PROP_CRTC_X;
|
|
} else if (strcmp(name, "CRTC_Y") == 0) {
|
|
return LIFTOFF_PROP_CRTC_Y;
|
|
} else if (strcmp(name, "CRTC_W") == 0) {
|
|
return LIFTOFF_PROP_CRTC_W;
|
|
} else if (strcmp(name, "CRTC_H") == 0) {
|
|
return LIFTOFF_PROP_CRTC_H;
|
|
} else if (strcmp(name, "zpos") == 0) {
|
|
return LIFTOFF_PROP_ZPOS;
|
|
}
|
|
return -1;
|
|
}
|
|
|
|
struct liftoff_display *liftoff_display_create(int drm_fd)
|
|
{
|
|
struct liftoff_display *display;
|
|
drmModeRes *drm_res;
|
|
drmModePlaneRes *drm_plane_res;
|
|
uint32_t i;
|
|
|
|
display = calloc(1, sizeof(*display));
|
|
if (display == NULL) {
|
|
liftoff_log_errno(LIFTOFF_ERROR, "calloc");
|
|
return NULL;
|
|
}
|
|
|
|
liftoff_list_init(&display->planes);
|
|
liftoff_list_init(&display->outputs);
|
|
|
|
display->drm_fd = dup(drm_fd);
|
|
if (display->drm_fd < 0) {
|
|
liftoff_log_errno(LIFTOFF_ERROR, "dup");
|
|
liftoff_display_destroy(display);
|
|
return NULL;
|
|
}
|
|
|
|
drm_res = drmModeGetResources(drm_fd);
|
|
if (drm_res == NULL) {
|
|
liftoff_log_errno(LIFTOFF_ERROR, "drmModeGetResources");
|
|
liftoff_display_destroy(display);
|
|
return NULL;
|
|
}
|
|
|
|
display->crtcs = malloc(drm_res->count_crtcs * sizeof(uint32_t));
|
|
if (display->crtcs == NULL) {
|
|
liftoff_log_errno(LIFTOFF_ERROR, "malloc");
|
|
drmModeFreeResources(drm_res);
|
|
liftoff_display_destroy(display);
|
|
return NULL;
|
|
}
|
|
display->crtcs_len = drm_res->count_crtcs;
|
|
memcpy(display->crtcs, drm_res->crtcs,
|
|
drm_res->count_crtcs * sizeof(uint32_t));
|
|
|
|
drmModeFreeResources(drm_res);
|
|
|
|
/* TODO: allow users to choose which layers to hand over */
|
|
drm_plane_res = drmModeGetPlaneResources(drm_fd);
|
|
if (drm_plane_res == NULL) {
|
|
liftoff_log_errno(LIFTOFF_ERROR, "drmModeGetPlaneResources");
|
|
liftoff_display_destroy(display);
|
|
return NULL;
|
|
}
|
|
|
|
for (i = 0; i < drm_plane_res->count_planes; i++) {
|
|
if (plane_create(display, drm_plane_res->planes[i]) == NULL) {
|
|
liftoff_display_destroy(display);
|
|
return NULL;
|
|
}
|
|
}
|
|
drmModeFreePlaneResources(drm_plane_res);
|
|
|
|
return display;
|
|
}
|
|
|
|
void liftoff_display_destroy(struct liftoff_display *display)
|
|
{
|
|
struct liftoff_plane *plane, *tmp;
|
|
|
|
close(display->drm_fd);
|
|
liftoff_list_for_each_safe(plane, tmp, &display->planes, link) {
|
|
plane_destroy(plane);
|
|
}
|
|
free(display->crtcs);
|
|
free(display);
|
|
}
|