mirror of
https://gitlab.freedesktop.org/emersion/libdisplay-info.git
synced 2024-12-25 21:59:08 +01:00
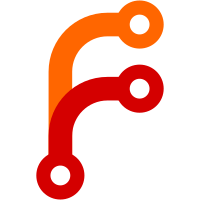
This will allow us to use the helpers from cta.c. The functions are marked "static inline" to avoid warnings about unused functions. Signed-off-by: Simon Ser <contact@emersion.fr>
39 lines
659 B
C
39 lines
659 B
C
#ifndef BITS_H
|
|
#define BITS_H
|
|
|
|
/**
|
|
* Utility functions to operate on bits.
|
|
*/
|
|
|
|
#include <stdbool.h>
|
|
#include <stddef.h>
|
|
#include <stdint.h>
|
|
|
|
/**
|
|
* Check whether a byte has a bit set.
|
|
*/
|
|
static inline bool
|
|
has_bit(uint8_t val, size_t index)
|
|
{
|
|
return val & (1 << index);
|
|
}
|
|
|
|
/**
|
|
* Extract a bit range from a byte.
|
|
*
|
|
* Both offsets are inclusive, start from zero, and high must be greater than low.
|
|
*/
|
|
static inline uint8_t
|
|
get_bit_range(uint8_t val, size_t high, size_t low)
|
|
{
|
|
size_t n;
|
|
uint8_t bitmask;
|
|
|
|
assert(high <= 7 && high >= low);
|
|
|
|
n = high - low + 1;
|
|
bitmask = (uint8_t) ((1 << n) - 1);
|
|
return (uint8_t) (val >> low) & bitmask;
|
|
}
|
|
|
|
#endif
|