mirror of
https://github.com/leozide/leocad
synced 2024-12-28 22:23:35 +01:00
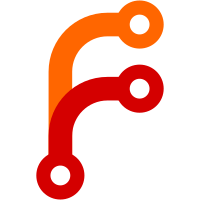
* Split synth info initialzation by type. We are going to remove the type enumeration and use a class hierarchy instead. This preparation will then be helpful. * Make Add...Parts() overrides of a virtual AddPart() function. Since we have a class hierarchy for the different synthesized pieces, we can now turn a case distinction into a virtual function call. * Move initialization based on type to derived class constructors. Move initialization of end transformations of flexible parts into class lcSynthInfoCurved. * Make GetDefaultControlPoints() virtual with overrides. * Remove obsolete enum lcSynthType. We have replaced its purpose by derived classes by now. * Initialize shock absorbers' spring part ID early. This removes the awkward early return that is needed in the if-else cascade. * Split lcSynthInfo into derived classes for curved and straight pieces. * Only curved parts have varying sections, start, middle, and end properties. Move the properties from the base class to the derived class that needs them. * Use derived classes to mark synthesized objects of different kinds. We will extend the derived classes in the upcoming commits. * PieceInfo is only needed to synthesize some hoses and shock absorbers. * Initialize edge part IDs of flexible hoses early. This removes another case distinction in AddParts(). * Verify the number of control points loaded from a model file. * Synthesize Technic universal joints. The direction of one end can be changed so that it points to the control point. * Technic universal joints need only the position of the control point. * Synthesize legacy universal joints.
51 lines
1.3 KiB
C++
51 lines
1.3 KiB
C++
#pragma once
|
|
|
|
#include "lc_math.h"
|
|
#include "piece.h"
|
|
|
|
class lcLibraryMeshData;
|
|
|
|
class lcSynthInfo
|
|
{
|
|
public:
|
|
explicit lcSynthInfo(float Length);
|
|
virtual ~lcSynthInfo() = default;
|
|
|
|
bool CanAddControlPoints() const
|
|
{
|
|
return mCurve;
|
|
}
|
|
|
|
bool IsCurve() const
|
|
{
|
|
return mCurve;
|
|
}
|
|
|
|
bool IsUnidirectional() const
|
|
{
|
|
return mUnidirectional;
|
|
}
|
|
|
|
bool IsNondirectional() const
|
|
{
|
|
return mNondirectional;
|
|
}
|
|
|
|
virtual void GetDefaultControlPoints(lcArray<lcPieceControlPoint>& ControlPoints) const = 0;
|
|
virtual void VerifyControlPoints(lcArray<lcPieceControlPoint>& ControlPoints) const = 0;
|
|
int InsertControlPoint(lcArray<lcPieceControlPoint>& ControlPoints, const lcVector3& Start, const lcVector3& End) const;
|
|
lcMesh* CreateMesh(const lcArray<lcPieceControlPoint>& ControlPoints) const;
|
|
|
|
protected:
|
|
using SectionCallbackFunc = std::function<void(const lcVector3& CurvePoint, int SegmentIndex, float t)>;
|
|
virtual void CalculateSections(const lcArray<lcPieceControlPoint>& ControlPoints, lcArray<lcMatrix44>& Sections, SectionCallbackFunc SectionCallback) const = 0;
|
|
virtual void AddParts(lcMemFile& File, lcLibraryMeshData& MeshData, const lcArray<lcMatrix44>& Sections) const = 0;
|
|
|
|
bool mCurve = false;
|
|
bool mUnidirectional = false;
|
|
bool mNondirectional = false;
|
|
float mLength;
|
|
};
|
|
|
|
void lcSynthInit();
|
|
|