mirror of
https://github.com/facundoolano/jorge.git
synced 2024-11-17 07:48:32 +01:00
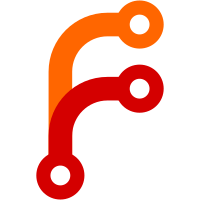
Squashed commit of the following: commit 0ee8a385f111be807b4485b42316df6698d962f9 Author: facundoolano <facundo.olano@gmail.com> Date: Mon Feb 12 14:12:22 2024 -0300 load layouts commit 1c2594bb8aa6d6d9fbafcb530fdcdbdec2c146e7 Author: facundoolano <facundo.olano@gmail.com> Date: Mon Feb 12 13:17:28 2024 -0300 add Site struct, explore some refactors commit 3d8acb3957f5ba38a6e48d2614b9af65f1219298 Author: facundoolano <facundo.olano@gmail.com> Date: Mon Feb 12 11:33:19 2024 -0300 prepare new phases structure for build command commit fe7dcf9fb08c7b3e5679cf08c80beecc2eeb36e5 Author: facundoolano <facundo.olano@gmail.com> Date: Mon Feb 12 10:57:52 2024 -0300 set template type commit d9faa70c8d2d23c9b62904e7429a82437517b9c5 Author: facundoolano <facundo.olano@gmail.com> Date: Mon Feb 12 10:49:30 2024 -0300 add Type to template commit 27e0feede10f6c1340ce722085011a5eebcaee13 Author: facundoolano <facundo.olano@gmail.com> Date: Sun Feb 11 19:01:16 2024 -0300 stub build and render extensions commit e25518de3440cb3df2aa5674523128eff1d23404 Author: facundoolano <facundo.olano@gmail.com> Date: Sun Feb 11 17:37:30 2024 -0300 pass pre-parsed layouts by arg instead commit b3c2c9ebeb0d07d425f6db6a1bbbb5ee61c04548 Author: facundoolano <facundo.olano@gmail.com> Date: Sun Feb 11 15:13:17 2024 -0300 first stab at recursively populating layouts commit 4fe112694a3c44056f7aa5bd2be0794abcf4aa2a Author: facundoolano <facundo.olano@gmail.com> Date: Sun Feb 11 14:33:07 2024 -0300 initial support for page bindings
182 lines
3.8 KiB
Go
182 lines
3.8 KiB
Go
package commands
|
|
|
|
import (
|
|
"fmt"
|
|
"github.com/facundoolano/blorg/templates"
|
|
"io"
|
|
"io/fs"
|
|
"os"
|
|
"path/filepath"
|
|
"strings"
|
|
)
|
|
|
|
const SRC_DIR = "src"
|
|
const TARGET_DIR = "target"
|
|
const LAYOUT_DIR = "layouts"
|
|
const FILE_RW_MODE = 0777
|
|
|
|
func Init() error {
|
|
// get working directory
|
|
// default to .
|
|
// if not exist, create directory
|
|
// copy over default files
|
|
fmt.Println("not implemented yet")
|
|
return nil
|
|
}
|
|
|
|
// Read the files in src/ render them and copy the result to target/
|
|
// FIXME pass src and target by arg
|
|
func Build() error {
|
|
_, err := os.ReadDir(SRC_DIR)
|
|
if os.IsNotExist(err) {
|
|
return fmt.Errorf("missing %s directory", SRC_DIR)
|
|
} else if err != nil {
|
|
return fmt.Errorf("couldn't read %s", SRC_DIR)
|
|
}
|
|
|
|
site := Site{
|
|
layouts: make(map[string]templates.Template),
|
|
}
|
|
|
|
// FIXME these sound like they should be site methods too
|
|
PHASES := []func(*Site) error{
|
|
loadConfig,
|
|
loadLayouts,
|
|
loadTemplates,
|
|
writeTarget,
|
|
}
|
|
for _, phaseFun := range PHASES {
|
|
if err := phaseFun(&site); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
|
|
return err
|
|
}
|
|
|
|
func loadConfig(site *Site) error {
|
|
// context["config"]
|
|
return nil
|
|
}
|
|
|
|
func loadLayouts(site *Site) error {
|
|
files, err := os.ReadDir(LAYOUT_DIR)
|
|
if os.IsNotExist(err) {
|
|
return nil
|
|
} else if err != nil {
|
|
return err
|
|
}
|
|
|
|
for _, entry := range files {
|
|
if !entry.IsDir() {
|
|
filename := entry.Name()
|
|
path := filepath.Join(LAYOUT_DIR, filename)
|
|
templ, err := templates.Parse(path)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
layout_name := strings.TrimSuffix(filename, filepath.Ext(filename))
|
|
site.layouts[layout_name] = *templ
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
func loadTemplates(site *Site) error {
|
|
return filepath.WalkDir(SRC_DIR, func(path string, entry fs.DirEntry, err error) error {
|
|
if !entry.IsDir() {
|
|
templ, err := templates.Parse(path)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
switch templ.Type {
|
|
case templates.POST:
|
|
site.posts = append(site.posts, *templ)
|
|
case templates.PAGE:
|
|
site.pages = append(site.pages, *templ)
|
|
}
|
|
// TODO add tags
|
|
}
|
|
return nil
|
|
})
|
|
}
|
|
|
|
func writeTarget(site *Site) error {
|
|
// clear previous target contents
|
|
os.RemoveAll(TARGET_DIR)
|
|
os.Mkdir(TARGET_DIR, FILE_RW_MODE)
|
|
|
|
// walk the source directory, creating directories and files at the target dir
|
|
templIndex := site.templateIndex()
|
|
return filepath.WalkDir(SRC_DIR, func(path string, entry fs.DirEntry, err error) error {
|
|
subpath, _ := filepath.Rel(SRC_DIR, path)
|
|
targetPath := filepath.Join(TARGET_DIR, subpath)
|
|
|
|
if entry.IsDir() {
|
|
os.MkdirAll(targetPath, FILE_RW_MODE)
|
|
} else {
|
|
|
|
if templ, ok := templIndex[path]; ok {
|
|
// if a template was found at source, render it
|
|
content, err := site.render(templ)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
// write the file contents over to target at the same location
|
|
targetPath = strings.TrimSuffix(targetPath, filepath.Ext(targetPath)) + templ.Ext()
|
|
fmt.Println("writing ", targetPath)
|
|
return os.WriteFile(targetPath, []byte(content), FILE_RW_MODE)
|
|
} else {
|
|
// if a non template was found, copy file as is
|
|
fmt.Println("writing ", targetPath)
|
|
return copyFile(path, targetPath)
|
|
}
|
|
}
|
|
|
|
return nil
|
|
})
|
|
}
|
|
|
|
func copyFile(source string, target string) error {
|
|
// does this need to be so verbose?
|
|
srcFile, err := os.Open(source)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
defer srcFile.Close()
|
|
|
|
targetFile, _ := os.Create(target)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
defer targetFile.Close()
|
|
|
|
_, err = io.Copy(targetFile, srcFile)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
return targetFile.Sync()
|
|
}
|
|
|
|
func New() error {
|
|
// prompt for title
|
|
// slugify
|
|
// fail if file already exist
|
|
// create a new .org file with the slug
|
|
// add front matter and org options
|
|
fmt.Println("not implemented yet")
|
|
return nil
|
|
}
|
|
|
|
func Serve() error {
|
|
// build
|
|
// serve target with file server
|
|
// (later watch and live reload)
|
|
fmt.Println("not implemented yet")
|
|
return nil
|
|
}
|