mirror of
https://github.com/facundoolano/jorge.git
synced 2024-11-16 07:47:40 +01:00
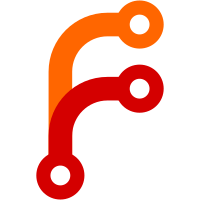
Squashed commit of the following: commit c45734422ef038438a0b6eb4c3065df9cbebcc5e Author: facundoolano <facundo.olano@gmail.com> Date: Tue Feb 13 21:27:47 2024 -0300 tags test passing commit 88294405603b2103f9f8cba3b130ad2d40e58a36 Author: facundoolano <facundo.olano@gmail.com> Date: Tue Feb 13 21:12:08 2024 -0300 ensure posts are sorted by date commit df7c945fcc1490613b6068da3fc487b9ffa44ba5 Author: facundoolano <facundo.olano@gmail.com> Date: Tue Feb 13 20:55:51 2024 -0300 prepare to test tags commit 3d7c3f380c3933d6cd9f949d3d2cf9ef144bd6ae Author: facundoolano <facundo.olano@gmail.com> Date: Tue Feb 13 20:51:08 2024 -0300 preload context in site fields commit a3afaacc67a9d748cfd42ecbeb7b2d302c3560b2 Author: facundoolano <facundo.olano@gmail.com> Date: Tue Feb 13 20:39:42 2024 -0300 test passing pending refactor commit 13de5017f654c8d82b1ba7beb01066a9c22f19fc Author: facundoolano <facundo.olano@gmail.com> Date: Tue Feb 13 20:18:07 2024 -0300 add (failing) archive test
108 lines
2.3 KiB
Go
108 lines
2.3 KiB
Go
package commands
|
|
|
|
import (
|
|
"fmt"
|
|
"github.com/facundoolano/blorg/site"
|
|
"io"
|
|
"io/fs"
|
|
"os"
|
|
"path/filepath"
|
|
"strings"
|
|
)
|
|
|
|
const SRC_DIR = "src"
|
|
const TARGET_DIR = "target"
|
|
const LAYOUTS_DIR = "layouts"
|
|
const FILE_RW_MODE = 0777
|
|
|
|
func Init() error {
|
|
// get working directory
|
|
// default to .
|
|
// if not exist, create directory
|
|
// copy over default files
|
|
fmt.Println("not implemented yet")
|
|
return nil
|
|
}
|
|
|
|
// Read the files in src/ render them and copy the result to target/
|
|
// TODO add root dir override support
|
|
func Build() error {
|
|
site, err := site.Load(SRC_DIR, LAYOUTS_DIR)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
// clear previous target contents
|
|
os.RemoveAll(TARGET_DIR)
|
|
os.Mkdir(TARGET_DIR, FILE_RW_MODE)
|
|
|
|
// walk the source directory, creating directories and files at the target dir
|
|
return filepath.WalkDir(SRC_DIR, func(path string, entry fs.DirEntry, err error) error {
|
|
subpath, _ := filepath.Rel(SRC_DIR, path)
|
|
targetPath := filepath.Join(TARGET_DIR, subpath)
|
|
|
|
if entry.IsDir() {
|
|
os.MkdirAll(targetPath, FILE_RW_MODE)
|
|
} else {
|
|
|
|
if templ, ok := site.Templates[path]; ok {
|
|
// if a template was found at source, render it
|
|
content, err := site.Render(templ)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
// write the file contents over to target at the same location
|
|
targetPath = strings.TrimSuffix(targetPath, filepath.Ext(targetPath)) + templ.Ext()
|
|
fmt.Println("writing", targetPath)
|
|
return os.WriteFile(targetPath, []byte(content), FILE_RW_MODE)
|
|
} else {
|
|
// if a non template was found, copy file as is
|
|
fmt.Println("writing", targetPath)
|
|
return copyFile(path, targetPath)
|
|
}
|
|
}
|
|
|
|
return nil
|
|
})
|
|
}
|
|
|
|
func copyFile(source string, target string) error {
|
|
// does this need to be so verbose?
|
|
srcFile, err := os.Open(source)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
defer srcFile.Close()
|
|
|
|
targetFile, _ := os.Create(target)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
defer targetFile.Close()
|
|
|
|
_, err = io.Copy(targetFile, srcFile)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
return targetFile.Sync()
|
|
}
|
|
|
|
func New() error {
|
|
// prompt for title
|
|
// slugify
|
|
// fail if file already exist
|
|
// create a new .org file with the slug
|
|
// add front matter and org options
|
|
fmt.Println("not implemented yet")
|
|
return nil
|
|
}
|
|
|
|
func Serve() error {
|
|
// build
|
|
// serve target with file server
|
|
// (later watch and live reload)
|
|
fmt.Println("not implemented yet")
|
|
return nil
|
|
}
|