mirror of
git://git.savannah.nongnu.org/eliot.git
synced 2024-11-17 07:48:27 +01:00
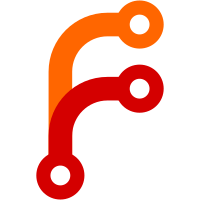
There are too many change to list properly, here is an overview of the main changes: - the dictionary is now in C++ - the dictionary has a new format, where it is possible to specify the letters, their points, their frequency, ... It is backwards compatible. - Eliot now supports non-ASCII characters everywhere - i18n of the compdic, listdic, regexpmain binaries - i18n of the wxWidgets interface (now in english by default)
202 lines
5 KiB
C++
202 lines
5 KiB
C++
/*****************************************************************************
|
|
* Eliot
|
|
* Copyright (C) 2005-2007 Antoine Fraboulet & Olivier Teulière
|
|
* Authors: Antoine Fraboulet <antoine.fraboulet @@ free.fr>
|
|
* Olivier Teulière <ipkiss @@ gmail.com>
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA
|
|
*****************************************************************************/
|
|
|
|
/**
|
|
* \file history.cpp
|
|
* \brief Game history system
|
|
* \author Antoine Fraboulet
|
|
* \date 2005
|
|
*/
|
|
|
|
#include <string>
|
|
#include "rack.h"
|
|
#include "pldrack.h"
|
|
#include "move.h"
|
|
#include "turn.h"
|
|
#include "history.h"
|
|
#include "encoding.h"
|
|
#include "debug.h"
|
|
|
|
|
|
/* ******************************************************** */
|
|
/* ******************************************************** */
|
|
/* ******************************************************** */
|
|
|
|
|
|
History::History()
|
|
{
|
|
Turn* t = new Turn();
|
|
m_history.clear();
|
|
m_history.push_back(t);
|
|
}
|
|
|
|
|
|
History::~History()
|
|
{
|
|
for (unsigned int i = 0; i < m_history.size(); i++)
|
|
{
|
|
delete m_history[i];
|
|
}
|
|
}
|
|
|
|
|
|
unsigned int History::getSize() const
|
|
{
|
|
ASSERT(!m_history.empty(), "Invalid history size");
|
|
return m_history.size() - 1;
|
|
}
|
|
|
|
|
|
const PlayedRack& History::getCurrentRack() const
|
|
{
|
|
return m_history.back()->getPlayedRack();
|
|
}
|
|
|
|
|
|
void History::setCurrentRack(const PlayedRack &iPld)
|
|
{
|
|
m_history.back()->setPlayedRack(iPld);
|
|
}
|
|
|
|
|
|
const Turn& History::getPreviousTurn() const
|
|
{
|
|
int idx = m_history.size() - 2;
|
|
ASSERT(0 <= idx , "No previous turn");
|
|
return *(m_history[idx]);
|
|
}
|
|
|
|
|
|
const Turn& History::getTurn(unsigned int n) const
|
|
{
|
|
ASSERT(n < m_history.size(), "Wrong turn number");
|
|
return *(m_history[n]);
|
|
}
|
|
|
|
|
|
bool History::beforeFirstRound() const
|
|
{
|
|
for (unsigned int i = 0; i < m_history.size() - 1; i++)
|
|
{
|
|
if (m_history[i]->getMove().getType() == Move::VALID_ROUND)
|
|
return false;
|
|
}
|
|
return true;
|
|
}
|
|
|
|
|
|
void History::playMove(unsigned int iPlayer, unsigned int iTurn, const Move &iMove)
|
|
{
|
|
Turn * current_turn = m_history.back();
|
|
|
|
// Set the number and the round
|
|
current_turn->setNum(iTurn);
|
|
current_turn->setPlayer(iPlayer);
|
|
current_turn->setMove(iMove);
|
|
|
|
// Get what was the rack for the current turn
|
|
Rack rack;
|
|
current_turn->getPlayedRack().getRack(rack);
|
|
|
|
if (iMove.getType() == Move::VALID_ROUND)
|
|
{
|
|
// Remove the played tiles from the rack
|
|
const Round &round = iMove.getRound();
|
|
for (unsigned int i = 0; i < round.getWordLen(); i++)
|
|
{
|
|
if (round.isPlayedFromRack(i))
|
|
{
|
|
if (round.isJoker(i))
|
|
rack.remove(Tile::Joker());
|
|
else
|
|
rack.remove(round.getTile(i));
|
|
}
|
|
}
|
|
}
|
|
else if (iMove.getType() == Move::CHANGE_LETTERS)
|
|
{
|
|
// Remove the changed tiles from the rack
|
|
const wstring & changed = iMove.getChangedLetters();
|
|
for (unsigned int i = 0; i < changed.size(); ++i)
|
|
{
|
|
rack.remove(Tile(changed[i]));
|
|
}
|
|
}
|
|
|
|
// Create a new turn
|
|
Turn * next_turn = new Turn();
|
|
PlayedRack pldrack;
|
|
pldrack.setOld(rack);
|
|
next_turn->setPlayedRack(pldrack);
|
|
m_history.push_back(next_turn);
|
|
}
|
|
|
|
|
|
void History::removeLastTurn()
|
|
{
|
|
int idx = m_history.size();
|
|
ASSERT(0 < idx , "Wrong turn number");
|
|
|
|
if (idx > 1)
|
|
{
|
|
Turn *t = m_history.back();
|
|
m_history.pop_back();
|
|
delete t;
|
|
}
|
|
|
|
// Now we have the previous played round in back()
|
|
Turn *t = m_history.back();
|
|
t->setNum(0);
|
|
t->setPlayer(0);
|
|
//t->setRound(Round());
|
|
#ifdef BACK_REMOVE_RACK_NEW_PART
|
|
t->getPlayedRound().setNew(Rack());
|
|
#endif
|
|
}
|
|
|
|
|
|
wstring History::toString() const
|
|
{
|
|
wstring rs;
|
|
#ifdef DEBUG
|
|
wchar_t buff[5];
|
|
_swprintf(buff, 4, L"%ld", m_history.size());
|
|
rs = L"history size = " + wstring(buff) + L"\n\n";
|
|
#endif
|
|
for (unsigned int i = 0; i < m_history.size(); i++)
|
|
{
|
|
Turn *t = m_history[i];
|
|
rs += t->toString() + L"\n";
|
|
}
|
|
return rs;
|
|
}
|
|
|
|
/* ******************************************************** */
|
|
/* ******************************************************** */
|
|
/* ******************************************************** */
|
|
|
|
|
|
/// Local Variables:
|
|
/// mode: c++
|
|
/// mode: hs-minor
|
|
/// c-basic-offset: 4
|
|
/// indent-tabs-mode: nil
|
|
/// End:
|