mirror of
https://gitlab.com/c3d/db48x.git
synced 2024-09-29 05:36:58 +02:00
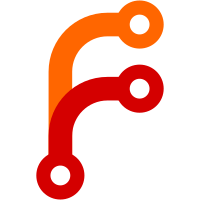
The `QSettings` class is only used to store the path to the actual state. The use of the file dialog seems to cause some trouble with the current working directory, and this impacts the display of the help file. Signed-off-by: Christophe de Dinechin <christophe@dinechin.org>
111 lines
2.8 KiB
C++
111 lines
2.8 KiB
C++
#ifndef SIM_WINDOW_H
|
|
#define SIM_WINDOW_H
|
|
// ****************************************************************************
|
|
// sim-window.h DB48X project
|
|
// ****************************************************************************
|
|
//
|
|
// File Description:
|
|
//
|
|
// Main window of the simulator
|
|
//
|
|
//
|
|
//
|
|
//
|
|
//
|
|
//
|
|
//
|
|
//
|
|
// ****************************************************************************
|
|
// (C) 2022 Christophe de Dinechin <christophe@dinechin.org>
|
|
// This software is licensed under the terms outlined in LICENSE.txt
|
|
// ****************************************************************************
|
|
// This file is part of DB48X.
|
|
//
|
|
// DB48X is free software: you can redistribute it and/or modify
|
|
// it under the terms outlined in the LICENSE.txt file
|
|
//
|
|
// DB48X is distributed in the hope that it will be useful,
|
|
// but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
|
|
// ****************************************************************************
|
|
|
|
#include "sim-rpl.h"
|
|
#include "tests.h"
|
|
#include "ui_sim-window.h"
|
|
|
|
#include <QAbstractEventDispatcher>
|
|
#include <QFile>
|
|
#include <QMainWindow>
|
|
|
|
|
|
class TestsThread : public QThread
|
|
// ----------------------------------------------------------------------------
|
|
// A thread to run the automated tests
|
|
// ----------------------------------------------------------------------------
|
|
{
|
|
public:
|
|
TestsThread(QObject *parent): QThread(parent), onlyCurrent() {}
|
|
~TestsThread()
|
|
{
|
|
if (isRunning())
|
|
while (isFinished())
|
|
terminate();
|
|
}
|
|
void run()
|
|
{
|
|
tests TestSuite;
|
|
TestSuite.run(onlyCurrent);
|
|
}
|
|
bool onlyCurrent;
|
|
};
|
|
|
|
|
|
class Highlight : public QWidget
|
|
{
|
|
Q_OBJECT;
|
|
public:
|
|
Highlight(QWidget *parent): QWidget(parent) {}
|
|
void paintEvent(QPaintEvent *);
|
|
|
|
public slots:
|
|
void keyResizeSlot(const QRect &rect);
|
|
};
|
|
|
|
class MainWindow : public QMainWindow
|
|
{
|
|
Q_OBJECT;
|
|
|
|
Ui::MainWindow ui;
|
|
RPLThread rpl;
|
|
TestsThread tests;
|
|
|
|
public:
|
|
explicit MainWindow(QWidget *parent = 0);
|
|
~MainWindow();
|
|
|
|
void pushKey(int key);
|
|
static MainWindow * theMainWindow() { return mainWindow; }
|
|
|
|
protected:
|
|
virtual void keyPressEvent(QKeyEvent *ev);
|
|
virtual void keyReleaseEvent(QKeyEvent *ev);
|
|
bool eventFilter(QObject *obj, QEvent *ev);
|
|
void resizeEvent(QResizeEvent *event);
|
|
|
|
protected:
|
|
static MainWindow *mainWindow;
|
|
Highlight *highlight;
|
|
|
|
signals:
|
|
void keyResizeSignal(const QRect &rect);
|
|
|
|
};
|
|
|
|
|
|
template <typename F>
|
|
static void postToThread(F && fun, QThread *thread = qApp->thread()) {
|
|
auto *obj = QAbstractEventDispatcher::instance(thread);
|
|
Q_ASSERT(obj);
|
|
QMetaObject::invokeMethod(obj, std::forward<F>(fun));
|
|
}
|
|
#endif // SIM_WINDOW_H
|