mirror of
https://gitlab.com/fbb-git/cppannotations
synced 2024-11-16 07:48:44 +01:00
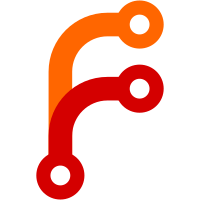
Annotations. The branches and tags directory are empty, since I couldn't svnadmin import a repostitory dump. Many earlier versions exist, though, and if you want the full archive, just let me know and I'll send you the svnadmin dump of my full C++ Annotations archive. Frank B. Brokken <f.b.brokken@rug.nl> git-svn-id: https://cppannotations.svn.sourceforge.net/svnroot/cppannotations/trunk@3 f6dd340e-d3f9-0310-b409-bdd246841980
48 lines
1.1 KiB
C++
48 lines
1.1 KiB
C++
#include <functional>
|
|
#include <vector>
|
|
#include <algorithm>
|
|
#include <iostream>
|
|
#include <string>
|
|
#include <cctype>
|
|
#include <iterator>
|
|
|
|
class Caps
|
|
{
|
|
public:
|
|
std::string operator()(std::string const &src)
|
|
{
|
|
std::string tmp = src;
|
|
|
|
transform(tmp.begin(), tmp.end(), tmp.begin(), toupper);
|
|
return tmp;
|
|
}
|
|
};
|
|
|
|
using namespace std;
|
|
|
|
int main()
|
|
{
|
|
string words[] = {"alpha", "bravo", "charley"};
|
|
|
|
copy(words, transform(words, words + 3, words, Caps()),
|
|
ostream_iterator<string>(cout, " "));
|
|
cout << endl;
|
|
|
|
int values[] = {1, 2, 3, 4, 5};
|
|
vector<int> squares;
|
|
|
|
transform(values, values + 5, values,
|
|
back_inserter(squares), multiplies<int>());
|
|
|
|
copy(squares.begin(), squares.end(),
|
|
ostream_iterator<int>(cout, " "));
|
|
cout << endl;
|
|
|
|
return 0;
|
|
}
|
|
/*
|
|
Generated output:
|
|
|
|
ALPHA BRAVO CHARLEY
|
|
1 4 9 16 25
|
|
*/
|