mirror of
https://github.com/awesomeWM/awesome
synced 2024-11-17 07:47:41 +01:00
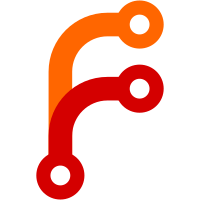
example config: graph gr_cpu { width = 80 height = "0.8" fg = "#336633" bg = "#000000" bordercolor = "#669966" padding_left = 0 mouse = {...} x = ... y = ... } Looks like here: http://www.calmar.ws/tmp/112-Sun-screen.png I renamed lpadding to padding_left, and bcolor to bordercolor also on the progressbar widget. The awesomerc page would still be to write, when this patch will get accepted. Hints are always welcomed.
173 lines
4.3 KiB
C
173 lines
4.3 KiB
C
/*
|
|
* widget.c - widget managing
|
|
*
|
|
* Copyright © 2007 Julien Danjou <julien@danjou.info>
|
|
* Copyright © 2007 Aldo Cortesi <aldo@nullcube.com>
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License along
|
|
* with this program; if not, write to the Free Software Foundation, Inc.,
|
|
* 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA.
|
|
*
|
|
*/
|
|
|
|
#include "util.h"
|
|
#include "widget.h"
|
|
#include "statusbar.h"
|
|
#include "event.h"
|
|
|
|
extern AwesomeConf globalconf;
|
|
|
|
const NameFuncLink WidgetList[] =
|
|
{
|
|
{"taglist", taglist_new},
|
|
{"layoutinfo", layoutinfo_new},
|
|
{"focustitle", focustitle_new},
|
|
{"textbox", textbox_new},
|
|
{"iconbox", iconbox_new},
|
|
{"netwmicon", netwmicon_new},
|
|
{"progressbar", progressbar_new},
|
|
{"graph", graph_new},
|
|
{"tasklist", tasklist_new},
|
|
{NULL, NULL}
|
|
};
|
|
|
|
void
|
|
widget_calculate_alignments(Widget *widget)
|
|
{
|
|
for(; widget; widget = widget->next)
|
|
{
|
|
if(widget->alignment == AlignFlex)
|
|
{
|
|
widget = widget->next;
|
|
break;
|
|
}
|
|
widget->alignment = AlignLeft;
|
|
}
|
|
|
|
if(widget)
|
|
for(; widget; widget = widget->next)
|
|
{
|
|
if (widget->alignment == AlignFlex)
|
|
warn("Multiple flex widgets in panel -"
|
|
" ignoring flex for all but the first.");
|
|
widget->alignment = AlignRight;
|
|
}
|
|
}
|
|
|
|
int
|
|
widget_calculate_offset(int barwidth, int widgetwidth, int offset, int alignment)
|
|
{
|
|
switch(alignment)
|
|
{
|
|
case AlignLeft:
|
|
case AlignFlex:
|
|
return offset;
|
|
}
|
|
return barwidth - offset - widgetwidth;
|
|
}
|
|
|
|
static Widget *
|
|
widget_find(char *name, int screen)
|
|
{
|
|
Widget *widget;
|
|
Statusbar *sb;
|
|
|
|
for(sb = globalconf.screens[screen].statusbar; sb; sb = sb->next)
|
|
for(widget = sb->widgets; widget; widget = widget->next)
|
|
if(a_strcmp(name, widget->name) == 0)
|
|
return widget;
|
|
|
|
return NULL;
|
|
}
|
|
|
|
static void
|
|
widget_common_button_press(Widget *widget, XButtonPressedEvent *ev)
|
|
{
|
|
Button *b;
|
|
|
|
for(b = widget->buttons; b; b = b->next)
|
|
if(ev->button == b->button && CLEANMASK(ev->state) == b->mod && b->func)
|
|
b->func(widget->statusbar->screen, b->arg);
|
|
}
|
|
|
|
static void
|
|
widget_common_tell(Widget *widget, char *command __attribute__ ((unused)))
|
|
{
|
|
warn("%s widget does not accept commands.\n", widget->name);
|
|
}
|
|
|
|
void
|
|
widget_common_new(Widget *widget, Statusbar *statusbar, cfg_t* config)
|
|
{
|
|
const char *name;
|
|
|
|
widget->statusbar = statusbar;
|
|
name = cfg_title(config);
|
|
widget->name = a_strdup(name);
|
|
widget->tell = widget_common_tell;
|
|
widget->button_press = widget_common_button_press;
|
|
widget->area.x = cfg_getint(config, "x");
|
|
widget->area.y = cfg_getint(config, "y");
|
|
widget->user_supplied_x = (widget->area.x != (int) 0xffffffff);
|
|
widget->user_supplied_y = (widget->area.y != (int) 0xffffffff);
|
|
}
|
|
|
|
/** Send command to widget
|
|
* \param screen Screen ID
|
|
* \param arg Widget command. Syntax depends on specific widget.
|
|
* \ingroup ui_callback
|
|
*/
|
|
void
|
|
uicb_widget_tell(int screen, char *arg)
|
|
{
|
|
Widget *widget;
|
|
char *p, *command;
|
|
int len;
|
|
|
|
if (!arg)
|
|
{
|
|
warn("Must specify a widget.\n");
|
|
return;
|
|
}
|
|
|
|
len = strlen(arg);
|
|
p = strtok(arg, " ");
|
|
if (!p)
|
|
{
|
|
warn("Ignoring malformed widget command.\n");
|
|
return;
|
|
}
|
|
|
|
widget = widget_find(p, screen);
|
|
if (!widget)
|
|
{
|
|
warn("No such widget: %s\n", p);
|
|
return;
|
|
}
|
|
|
|
if (p+strlen(p) < arg+len)
|
|
{
|
|
p = p + strlen(p) + 1;
|
|
command = p_new(char, strlen(p)+1);
|
|
strncpy(command, p, strlen(p));
|
|
widget->tell(widget, command);
|
|
p_delete(&command);
|
|
}
|
|
else
|
|
widget->tell(widget, NULL);
|
|
|
|
statusbar_draw_all(screen);
|
|
return;
|
|
}
|
|
|
|
// vim: filetype=c:expandtab:shiftwidth=4:tabstop=8:softtabstop=4:encoding=utf-8:textwidth=80
|