mirror of
https://github.com/awesomeWM/awesome
synced 2024-11-17 07:47:41 +01:00
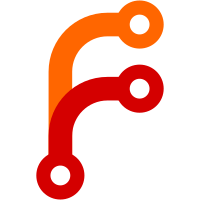
All versions of libxcb provide at least RandR 1.3 (if I remember correctly). With version 1.5, the concept of a monitor was added. This might make quite a difference for awesome and to help debugging bug reports that are due to missing support for monitors, the existence of this support was added to the --version output. However, many people feel like they are missing out due to this, even though the new RandR 1.5 stuff likely makes no difference for them. To help these people, instead of having a yes/no, just print the full RandR version. When a bug report that is due to missing monitor support shows up, we can look up if the reporter has RandR 1.5. All other people are no longer bothered. Example-reference: http://stackoverflow.com/questions/41773529/no-randr-1-5-support-in-test-install-of-awesome-4-0-what-to-check Signed-off-by: Uli Schlachter <psychon@znc.in>
97 lines
2.7 KiB
C
97 lines
2.7 KiB
C
/*
|
|
* version.c - version message utility functions
|
|
*
|
|
* Copyright © 2008 Julien Danjou <julien@danjou.info>
|
|
* Copyright © 2008 Hans Ulrich Niedermann <hun@n-dimensional.de>
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License along
|
|
* with this program; if not, write to the Free Software Foundation, Inc.,
|
|
* 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA.
|
|
*/
|
|
|
|
#include "config.h"
|
|
#include "common/version.h"
|
|
#include "awesome-version-internal.h"
|
|
|
|
#include <stdlib.h>
|
|
#include <stdio.h>
|
|
|
|
#include <lualib.h>
|
|
#include <lauxlib.h>
|
|
#include <xcb/randr.h> /* for XCB_RANDR_GET_MONITORS */
|
|
|
|
/** \brief Print version message and quit program.
|
|
* \param executable program name
|
|
*/
|
|
void
|
|
eprint_version(void)
|
|
{
|
|
lua_State *L = luaL_newstate();
|
|
luaL_openlibs(L);
|
|
lua_getglobal(L, "_VERSION");
|
|
lua_getglobal(L, "jit");
|
|
|
|
if (lua_istable(L, 2))
|
|
lua_getfield(L, 2, "version");
|
|
else
|
|
lua_pop(L, 1);
|
|
|
|
/* Either push version number or error message onto stack */
|
|
(void) luaL_dostring(L, "return require('lgi.version')");
|
|
|
|
#ifdef WITH_DBUS
|
|
const char *has_dbus = "✔";
|
|
#else
|
|
const char *has_dbus = "✘";
|
|
#endif
|
|
#ifdef HAS_EXECINFO
|
|
const char *has_execinfo = "✔";
|
|
#else
|
|
const char *has_execinfo = "✘";
|
|
#endif
|
|
|
|
printf("awesome %s (%s)\n"
|
|
" • Compiled against %s (running with %s)\n"
|
|
" • D-Bus support: %s\n"
|
|
" • execinfo support: %s\n"
|
|
" • xcb-randr version: %d.%d\n"
|
|
" • LGI version: %s\n",
|
|
AWESOME_VERSION, AWESOME_RELEASE,
|
|
LUA_RELEASE, lua_tostring(L, -2),
|
|
has_dbus, has_execinfo,
|
|
XCB_RANDR_MAJOR_VERSION, XCB_RANDR_MINOR_VERSION,
|
|
lua_tostring(L, -1));
|
|
lua_close(L);
|
|
|
|
exit(EXIT_SUCCESS);
|
|
}
|
|
|
|
/** Get version string.
|
|
* \return A string describing the current version.
|
|
*/
|
|
const char *
|
|
awesome_version_string(void)
|
|
{
|
|
return AWESOME_VERSION;
|
|
}
|
|
|
|
/** Get release string.
|
|
* \return A string describing the code name of the relase.
|
|
*/
|
|
const char *
|
|
awesome_release_string(void)
|
|
{
|
|
return AWESOME_RELEASE;
|
|
}
|
|
|
|
// vim: filetype=c:expandtab:shiftwidth=4:tabstop=8:softtabstop=4:textwidth=80
|