mirror of
https://github.com/awesomeWM/awesome
synced 2024-11-17 07:47:41 +01:00
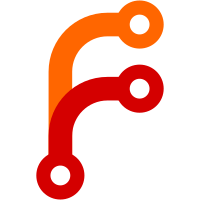
Loading a file normally has the same behaviour as before. First the cache is checked and if nothing is found, the file is loaded and cached. This commit changes the behaviour of loading a file uncached. This no longer removes the file from the cache if it is cached (why should it?) and also does not put it in the cache. This means that users of load_uncached and load_uncached_silently can now freely modify the resulting surface without interfering with other API users. Signed-off-by: Uli Schlachter <psychon@znc.in>
210 lines
7.1 KiB
Lua
210 lines
7.1 KiB
Lua
---------------------------------------------------------------------------
|
|
-- @author Uli Schlachter
|
|
-- @copyright 2012 Uli Schlachter
|
|
-- @release @AWESOME_VERSION@
|
|
-- @module gears.surface
|
|
---------------------------------------------------------------------------
|
|
|
|
local setmetatable = setmetatable
|
|
local type = type
|
|
local capi = { awesome = awesome }
|
|
local cairo = require("lgi").cairo
|
|
local color = nil
|
|
local gdebug = require("gears.debug")
|
|
|
|
-- Keep this in sync with build-utils/lgi-check.sh!
|
|
local ver_major, ver_minor, ver_patch = string.match(require('lgi.version'), '(%d)%.(%d)%.(%d)')
|
|
if tonumber(ver_major) <= 0 and (tonumber(ver_minor) < 7 or (tonumber(ver_minor) == 7 and tonumber(ver_patch) < 1)) then
|
|
error("lgi too old, need at least version 0.7.1")
|
|
end
|
|
|
|
local surface = { mt = {} }
|
|
local surface_cache = setmetatable({}, { __mode = 'v' })
|
|
|
|
local function get_default(arg)
|
|
if type(arg) == 'nil' then
|
|
return cairo.ImageSurface(cairo.Format.ARGB32, 0, 0)
|
|
end
|
|
return arg
|
|
end
|
|
|
|
--- Try to convert the argument into an lgi cairo surface.
|
|
-- This is usually needed for loading images by file name.
|
|
-- @param _surface The surface to load or nil
|
|
-- @param default The default value to return on error; when nil, then a surface
|
|
-- in an error state is returned.
|
|
-- @return The loaded surface, or the replacement default
|
|
-- @return An error message, or nil on success
|
|
function surface.load_uncached_silently(_surface, default)
|
|
local file
|
|
-- On nil, return some sane default
|
|
if not _surface then
|
|
return get_default(default)
|
|
end
|
|
-- lgi cairo surfaces don't get changed either
|
|
if cairo.Surface:is_type_of(_surface) then
|
|
return _surface
|
|
end
|
|
-- Strings are assumed to be file names and get loaded
|
|
if type(_surface) == "string" then
|
|
local err
|
|
file = _surface
|
|
_surface, err = capi.awesome.load_image(file)
|
|
if not _surface then
|
|
return get_default(default), err
|
|
end
|
|
end
|
|
-- Everything else gets forced into a surface
|
|
return cairo.Surface(_surface, true)
|
|
end
|
|
|
|
--- Try to convert the argument into an lgi cairo surface.
|
|
-- This is usually needed for loading images by file name and uses a cache.
|
|
-- In contrast to `load()`, errors are returned to the caller.
|
|
-- @param _surface The surface to load or nil
|
|
-- @param default The default value to return on error; when nil, then a surface
|
|
-- in an error state is returned.
|
|
-- @return The loaded surface, or the replacement default, or nil if called with
|
|
-- nil.
|
|
-- @return An error message, or nil on success
|
|
function surface.load_silently(_surface, default)
|
|
if type(_surface) == "string" then
|
|
local cache = surface_cache[_surface]
|
|
if cache then
|
|
return cache
|
|
end
|
|
local result, err = surface.load_uncached_silently(_surface, default)
|
|
if not err then
|
|
-- Cache the file
|
|
surface_cache[_surface] = result
|
|
end
|
|
return result, err
|
|
end
|
|
return surface.load_uncached_silently(_surface, default)
|
|
end
|
|
|
|
local function do_load_and_handle_errors(_surface, func)
|
|
if type(_surface) == 'nil' then
|
|
return get_default()
|
|
end
|
|
local result, err = func(_surface, false)
|
|
if result then
|
|
return result
|
|
end
|
|
gdebug.print_error("Failed to load '" .. tostring(_surface) .. "': " .. tostring(err))
|
|
return get_default()
|
|
end
|
|
|
|
--- Try to convert the argument into an lgi cairo surface.
|
|
-- This is usually needed for loading images by file name. Errors are handled
|
|
-- via `gears.debug.print_error`.
|
|
-- @param _surface The surface to load or nil
|
|
-- @return The loaded surface, or nil
|
|
function surface.load_uncached(_surface)
|
|
return do_load_and_handle_errors(_surface, surface.load_uncached_silently)
|
|
end
|
|
|
|
--- Try to convert the argument into an lgi cairo surface.
|
|
-- This is usually needed for loading images by file name. Errors are handled
|
|
-- via `gears.debug.print_error`.
|
|
-- @param _surface The surface to load or nil
|
|
-- @return The loaded surface, or nil
|
|
function surface.load(_surface)
|
|
return do_load_and_handle_errors(_surface, surface.load_silently)
|
|
end
|
|
|
|
function surface.mt.__call(_, ...)
|
|
return surface.load(...)
|
|
end
|
|
|
|
--- Get the size of a cairo surface
|
|
-- @param surf The surface you are interested in
|
|
-- @return The surface's width and height
|
|
function surface.get_size(surf)
|
|
local cr = cairo.Context(surf)
|
|
local x, y, w, h = cr:clip_extents()
|
|
return w - x, h - y
|
|
end
|
|
|
|
--- Create a copy of a cairo surface.
|
|
-- The surfaces returned by `surface.load` are cached and must not be
|
|
-- modified to avoid unintended side-effects. This function allows to create
|
|
-- a copy of a cairo surface. This copy can then be freely modified.
|
|
-- The surface returned will be as compatible as possible to the input
|
|
-- surface. For example, it will likely be of the same surface type as the
|
|
-- input. The details are explained in the `create_similar` function on a cairo
|
|
-- surface.
|
|
-- @param s Source surface.
|
|
-- @return The surface's duplicate.
|
|
function surface.duplicate_surface(s)
|
|
s = surface.load(s)
|
|
|
|
-- Figure out surface size (this does NOT work for unbounded recording surfaces)
|
|
local cr = cairo.Context(s)
|
|
local x, y, w, h = cr:clip_extents()
|
|
|
|
-- Create a copy
|
|
local result = s:create_similar(s.content, w - x, h - y)
|
|
cr = cairo.Context(result)
|
|
cr:set_source_surface(s, 0, 0)
|
|
cr.operator = cairo.Operator.SOURCE
|
|
cr:paint()
|
|
return result
|
|
end
|
|
|
|
--- Create a surface from a `gears.shape`
|
|
-- Any additional parameters will be passed to the shape function
|
|
-- @tparam number width The surface width
|
|
-- @tparam number height The surface height
|
|
-- @param shape A `gears.shape` compatible function
|
|
-- @param[opt=white] shape_color The shape color or pattern
|
|
-- @param[opt=transparent] bg_color The surface background color
|
|
-- @treturn cairo.surface the new surface
|
|
function surface.load_from_shape(width, height, shape, shape_color, bg_color, ...)
|
|
color = color or require("gears.color")
|
|
|
|
local img = cairo.ImageSurface(cairo.Format.ARGB32, width, height)
|
|
local cr = cairo.Context(img)
|
|
|
|
cr:set_source(color(bg_color or "#00000000"))
|
|
cr:paint()
|
|
|
|
cr:set_source(color(shape_color or "#000000"))
|
|
|
|
shape(cr, width, height, ...)
|
|
|
|
cr:fill()
|
|
|
|
return img
|
|
end
|
|
|
|
--- Apply a shape to a client or a wibox.
|
|
--
|
|
-- If the wibox or client size change, this function need to be called
|
|
-- again.
|
|
-- @param draw A wibox or a client
|
|
-- @param shape or gears.shape function or a custom function with a context,
|
|
-- width and height as parameter.
|
|
-- @param[opt] Any additional parameters will be passed to the shape function
|
|
function surface.apply_shape_bounding(draw, shape, ...)
|
|
local geo = draw:geometry()
|
|
|
|
local img = cairo.ImageSurface(cairo.Format.A1, geo.width, geo.height)
|
|
local cr = cairo.Context(img)
|
|
|
|
cr:set_operator(cairo.Operator.CLEAR)
|
|
cr:set_source_rgba(0,0,0,1)
|
|
cr:paint()
|
|
cr:set_operator(cairo.Operator.SOURCE)
|
|
cr:set_source_rgba(1,1,1,1)
|
|
|
|
shape(cr, geo.width, geo.height, ...)
|
|
|
|
cr:fill()
|
|
|
|
draw.shape_bounding = img._native
|
|
end
|
|
|
|
return setmetatable(surface, surface.mt)
|
|
|
|
-- vim: filetype=lua:expandtab:shiftwidth=4:tabstop=8:softtabstop=4:textwidth=80
|