mirror of
https://github.com/jezhiggins/arabica
synced 2024-12-27 21:58:30 +01:00
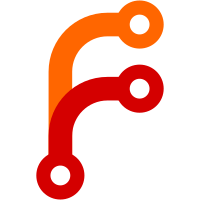
To ease implementing scan, BinaryExpression and UnaryExpression now inherit XPathExpression_impl. Other classes now derived only from Binary|UnaryExpression rather than from XPathExpression_impl as well.
124 lines
5.7 KiB
C++
124 lines
5.7 KiB
C++
#ifndef ARABICA_XPATHIC_XPATH_ARITHMETIC_HPP
|
|
#define ARABICA_XPATHIC_XPATH_ARITHMETIC_HPP
|
|
|
|
#include "xpath_value.hpp"
|
|
|
|
namespace Arabica
|
|
{
|
|
namespace XPath
|
|
{
|
|
namespace impl
|
|
{
|
|
|
|
template<class string_type, class string_adaptor>
|
|
class PlusOperator : public BinaryExpression<string_type, string_adaptor>
|
|
{
|
|
typedef BinaryExpression<string_type, string_adaptor> baseT;
|
|
public:
|
|
PlusOperator(XPathExpression_impl<string_type, string_adaptor>* lhs, XPathExpression_impl<string_type, string_adaptor>* rhs) :
|
|
BinaryExpression<string_type, string_adaptor>(lhs, rhs) { }
|
|
|
|
virtual ValueType type() const { return NUMBER; }
|
|
|
|
virtual XPathValue<string_type, string_adaptor> evaluate(const DOM::Node<string_type, string_adaptor>& context,
|
|
const ExecutionContext<string_type, string_adaptor>& executionContext) const
|
|
{
|
|
return NumericValue<string_type, string_adaptor>::createValue(baseT::lhs()->evaluateAsNumber(context, executionContext) + baseT::rhs()->evaluateAsNumber(context, executionContext));
|
|
} // evaluate
|
|
}; // class PlusOperator
|
|
|
|
template<class string_type, class string_adaptor>
|
|
class MinusOperator : public BinaryExpression<string_type, string_adaptor>
|
|
{
|
|
typedef BinaryExpression<string_type, string_adaptor> baseT;
|
|
public:
|
|
MinusOperator(XPathExpression_impl<string_type, string_adaptor>* lhs, XPathExpression_impl<string_type, string_adaptor>* rhs) :
|
|
BinaryExpression<string_type, string_adaptor>(lhs, rhs) { }
|
|
|
|
virtual ValueType type() const { return NUMBER; }
|
|
|
|
virtual XPathValue<string_type, string_adaptor> evaluate(const DOM::Node<string_type, string_adaptor>& context,
|
|
const ExecutionContext<string_type, string_adaptor>& executionContext) const
|
|
{
|
|
return NumericValue<string_type, string_adaptor>::createValue(baseT::lhs()->evaluateAsNumber(context, executionContext) - baseT::rhs()->evaluateAsNumber(context, executionContext));
|
|
} // evaluate
|
|
}; // class MinusOperator
|
|
|
|
template<class string_type, class string_adaptor>
|
|
class MultiplyOperator : public BinaryExpression<string_type, string_adaptor>
|
|
{
|
|
typedef BinaryExpression<string_type, string_adaptor> baseT;
|
|
public:
|
|
MultiplyOperator(XPathExpression_impl<string_type, string_adaptor>* lhs, XPathExpression_impl<string_type, string_adaptor>* rhs) :
|
|
BinaryExpression<string_type, string_adaptor>(lhs, rhs) { }
|
|
|
|
virtual ValueType type() const { return NUMBER; }
|
|
|
|
virtual XPathValue<string_type, string_adaptor> evaluate(const DOM::Node<string_type, string_adaptor>& context,
|
|
const ExecutionContext<string_type, string_adaptor>& executionContext) const
|
|
{
|
|
return NumericValue<string_type, string_adaptor>::createValue(baseT::lhs()->evaluateAsNumber(context, executionContext) * baseT::rhs()->evaluateAsNumber(context, executionContext));
|
|
} // evaluate
|
|
}; // class MultiplyOperator
|
|
|
|
template<class string_type, class string_adaptor>
|
|
class DivideOperator : public BinaryExpression<string_type, string_adaptor>
|
|
{
|
|
typedef BinaryExpression<string_type, string_adaptor> baseT;
|
|
public:
|
|
DivideOperator(XPathExpression_impl<string_type, string_adaptor>* lhs, XPathExpression_impl<string_type, string_adaptor>* rhs) :
|
|
BinaryExpression<string_type, string_adaptor>(lhs, rhs) { }
|
|
|
|
virtual ValueType type() const { return NUMBER; }
|
|
|
|
virtual XPathValue<string_type, string_adaptor> evaluate(const DOM::Node<string_type, string_adaptor>& context,
|
|
const ExecutionContext<string_type, string_adaptor>& executionContext) const
|
|
{
|
|
return NumericValue<string_type, string_adaptor>::createValue(baseT::lhs()->evaluateAsNumber(context, executionContext) / baseT::rhs()->evaluateAsNumber(context, executionContext));
|
|
} // evaluate
|
|
}; // class DivideOperator
|
|
|
|
template<class string_type, class string_adaptor>
|
|
class ModOperator : public BinaryExpression<string_type, string_adaptor>
|
|
{
|
|
typedef BinaryExpression<string_type, string_adaptor> baseT;
|
|
public:
|
|
ModOperator(XPathExpression_impl<string_type, string_adaptor>* lhs, XPathExpression_impl<string_type, string_adaptor>* rhs) :
|
|
BinaryExpression<string_type, string_adaptor>(lhs, rhs) { }
|
|
|
|
virtual ValueType type() const { return NUMBER; }
|
|
|
|
virtual XPathValue<string_type, string_adaptor> evaluate(const DOM::Node<string_type, string_adaptor>& context,
|
|
const ExecutionContext<string_type, string_adaptor>& executionContext) const
|
|
{
|
|
double l = baseT::lhs()->evaluateAsNumber(context, executionContext);
|
|
double r = baseT::rhs()->evaluateAsNumber(context, executionContext);
|
|
|
|
if(isNaN(l) || isNaN(r))
|
|
return NumericValue<string_type, string_adaptor>::createValue(NaN);
|
|
return NumericValue<string_type, string_adaptor>::createValue(static_cast<long>(l) % static_cast<long>(r));
|
|
} // evaluate
|
|
}; // class ModOperator
|
|
|
|
template<class string_type, class string_adaptor>
|
|
class UnaryNegative : public UnaryExpression<string_type, string_adaptor>
|
|
{
|
|
typedef UnaryExpression<string_type, string_adaptor> baseT;
|
|
public:
|
|
UnaryNegative(XPathExpression_impl<string_type, string_adaptor>* expr) :
|
|
UnaryExpression<string_type, string_adaptor>(expr) { }
|
|
|
|
virtual ValueType type() const { return NUMBER; }
|
|
|
|
virtual XPathValue<string_type, string_adaptor> evaluate(const DOM::Node<string_type, string_adaptor>& context,
|
|
const ExecutionContext<string_type, string_adaptor>& executionContext) const
|
|
{
|
|
return NumericValue<string_type, string_adaptor>::createValue(-baseT::expr()->evaluate(context, executionContext).asNumber());
|
|
} // evaluate
|
|
}; // class UnaryNegative
|
|
|
|
} // namespace impl
|
|
} // namespace XPath
|
|
} // namespace Arabica
|
|
|
|
#endif
|